UnBoundLocalError: local variable referenced before assignment (Python)
Solution 1
It's because you have assigned the variable servo_quadrant
under one of the preceding if
conditions in your function, and if none of the conditions return True, you will haven't any servo_quadrant
. For getting ride of this problem you need to initial this variable in your function.
You can put servo_quadrant = 0
on top level of your function or you can check the value of the servo_quadrant
before you return anything :
if servo_quadrant :
return servo_quadrant
return None
Also Note that you need to reassign variable servo_val
:
if servo_val < 0: servo_val=360 + servo_val
Demo:
def servo_to_quadrant(servo_val):
servo_quadrant=0
if servo_val < 0: servo_val=360 + servo_val
if servo_val >= 360: servo_val = servo_val - 360
if servo_val >= 0 and servo_val < 90: servo_quadrant = 1
if servo_val >= 90 and servo_val < 180: servo_quadrant = 2
if servo_val >= 180 and servo_val < 270: servo_quadrant = 3
if servo_val >= 270 and servo_val < 360: servo_quadrant = 4
return servo_quadrant
servo_val = -30
quadrant = servo_to_quadrant(servo_val)
print quadrant
Result:
4
Solution 2
This is because you try to modify servo_quadrant which isn't defined in your function. Python uses global scope by default if you just read a variable. So if you don't modify it everything will work fine. If you need to modify it just add global servo_quadrant
at the beginning of your function.
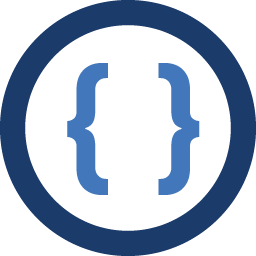
Admin
Updated on November 21, 2020Comments
-
Admin over 3 years
I'm trying to create a function
servo_to_quadrant
that returns the valueservo_quadrant
.Questions similar to this one have involved there being an issue with a global variable outside of the function. I don't think that's the issue in this case, as the variable is only needed from within the function (although I could be wrong).
Code:
def servo_to_quadrant(servo_val): if servo_val < 0: 360 + servo_val if servo_val >= 360: servo_val = servo_val - 360 if servo_val >= 0 and servo_val < 90: servo_quadrant = 1 if servo_val >= 90 and servo_val < 180: servo_quadrant = 2 if servo_val >= 180 and servo_val < 270: servo_quadrant = 3 if servo_val >= 270 and servo_val < 360: servo_quadrant = 4 return servo_quadrant servo_val = -30 quadrant = servo_to_quadrant(servo_val) print(quadrant)
Error:
Traceback (most recent call last): File "test2.py", line 11, in <module> quadrant = servo_to_quadrant(servo_val) File "test2.py", line 8, in servo_to_quadrant return servo_quadrant UnboundLocalError: local variable 'servo_quadrant' referenced before assignment