Uncaught (in promise) DOMException: Unable to decode audio data
14,213
I encountered the same error. Updating Chrome did not fix it. However, debugging in Firefox instead gave me a much more descriptive error:
The buffer passed to decodeAudioData contains an unknown content type.
Uncaught (in promise) DOMException: MediaDecodeAudioDataUnknownContentType
Uncaught (in promise) DOMException: The given encoding is not supported.
Which by the way was occurring because the .mp3 file I wanted to load wasn't found. So I was making a web request, receiving the 404 HTML page, and trying to load that as an mp3, which failed as an 'unsupported audio format'.
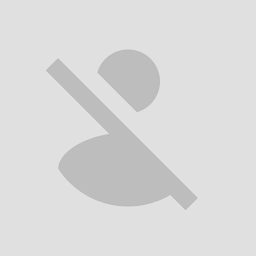
Author by
Aman Gupta
Updated on June 05, 2022Comments
-
Aman Gupta about 2 years
I'm having an issue with
decodeAudioData
method usingWeb Audio API
to playback in Chrome (it works fine in Firefox)-I am sending the audio buffer recorded by media recorder back from the server.
Server side
wss = new WebSocketServer({server: server}, function () {}); wss.on('connection', function connection(ws) { ws.binaryType = "arraybuffer"; ws.on('message', function incoming(message) { if ((typeof message) == 'string') { console.log("string message: ", message); } else { console.log("not string: ", message); ws.send(message); } }); });
Client side
window.AudioContext = window.AudioContext||window.webkitAudioContext; navigator.getUserMedia = (navigator.getUserMedia || navigator.webkitGetUserMedia || navigator.mozGetUserMedia || navigator.msGetUserMedia); var context = new AudioContext(); var mediaRecorder; var chunks = []; var startTime = 0; ws = new WebSocket(url); ws.binaryType = "arraybuffer"; ws.onmessage = function(message) { if (message.data instanceof ArrayBuffer) { context.decodeAudioData(message.data, function(soundBuffer){ playBuffer(soundBuffer); },function(x) { console.log("decoding failed", x) }); } else { console.log("not arrayBuffer", message.data); } }; createMediaRecorder(); function createMediaRecorder() { if (navigator.getUserMedia) { console.log('getUserMedia supported.'); var constraints = { "audio": true }; var onSuccess = function(stream) { var options = { audioBitsPerSecond : 128000, mimeType : 'audio/webm\;codecs=opus' }; mediaRecorder = new MediaRecorder(stream, options); }; var onError = function(err) { console.log('The following error occured: ' + err); }; navigator.getUserMedia(constraints, onSuccess, onError); } else { alert('getUserMedia not supported on your browser!'); } } function playBuffer(buf) { var source = context.createBufferSource(); source.buffer = buf; source.connect(context.destination); if (startTime == 0) startTime = context.currentTime + 0.1; // add 50ms latency to work well across systems source.start(startTime); startTime = startTime + source.buffer.duration; } function startRecording() { mediaRecorder.start(); getRecordedData(); } function getRecordedData() { mediaRecorder.ondataavailable = function(e) { console.log('ondataavailable: ', e.data); chunks.push(e.data); }; } function sendRecordedData() { var superBuffer = new Blob(chunks, {type: 'audio/ogg'}); ws.send(superBuffer); } function stopRecording() { mediaRecorder.stop(); mediaRecorder.onstop = function(e) { sendRecordedData(); chunks = []; }; }
While testing with firefox working fine but with chrome generate the following error:
Uncaught (in promise) DOMException: Unable to decode audio data
Any suggestion will be helpful, thanks in advance.