Uncaught TypeError: Object.values is not a function JavaScript
Solution 1
.values
is unsupported in many browsers - you can use .map
to get an array of all the values:
var vals = Object.keys(countries).map(function(key) {
return countries[key];
});
See MDN doc: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/values or Official doc: https://tc39.github.io/ecma262/#sec-object.values (thanks @evolutionxbox for correction)
Solution 2
It's also worth noting that only Node versions >= 7.0.0 fully support this.
Solution 3
For those who ended up here and are using Angular, adding import 'core-js/es7/object';
to polyfills.ts
file solved the problem for me.
/** IE9, IE10 and IE11 requires all of the following polyfills. **/
import 'core-js/es6/array';
import 'core-js/es6/date';
import 'core-js/es6/function';
import 'core-js/es6/map';
import 'core-js/es6/math';
import 'core-js/es6/number';
import 'core-js/es6/object';
import 'core-js/es6/parse-float';
import 'core-js/es6/parse-int';
import 'core-js/es6/regexp';
import 'core-js/es6/set';
import 'core-js/es6/string';
import 'core-js/es6/symbol';
import 'core-js/es6/weak-map';
import 'core-js/es7/array';
import 'core-js/es7/object'; // added import
Solution 4
Using "for...in" as discussed at mozilla: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_objects/Object/values
Here is the code I used:
function objectValues(obj) {
let vals = [];
for (const prop in obj) {
vals.push(obj[prop]);
}
return vals;
}
// usage
let obj = {k1: 'v1', k2: 'v1', k3: 'v1'};
console.log(objectValues(obj)); // prints the array [ 'v1', 'v1', 'v1' ]
// OR
console.log(objectValues(obj).join(', ')); // prints the string 'v1, v1, v1'
Solution 5
Looks like this issue is fixed in Safari latest version. I came around the same issue. This issue occurs in browser version 9.0.1 and does not occur in 10.1.1
Editing to add the attachments:
[snippet][1]
[object value][2]
[browser version][3]
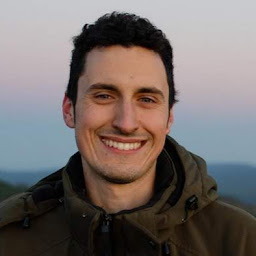
Alex Fallenstedt
I build web applications and mobile apps I am a senior software engineer who strives for test-driven development, translating business requirements into technical requirements, and an agile based development model. I consider these to be the primary elements for delivering quality and maintainable software with high-confidence. In the past, I was a lead photographer for a magazine in Portland, and operated a wedding photography business.
Updated on June 22, 2020Comments
-
Alex Fallenstedt almost 4 years
I have a simple object like the one below:
var countries = { "Argentina":1, "Canada":2, "Egypt":1, };
I need to create two arrays. The first array is an array of all the keys from the object. I created this array by:
var labels = Object.keys(countries);
This works well. I obtain an array of countries. Now when I try to create an array from the values...
var labels = Object.values(countries);
I get this error:
Uncaught TypeError: Object.values is not a function JavaScript
I don't know what I am doing wrong. I
console.log countries
before and after I declarelabels
and the object remains the same. How do I properly useObject.values()
?