Unexpected value ‘HttpClient’
22,863
Solution 1
You have to import
the module HttpClientModule
and reference that in the imports.
// reference the module
import { HttpClientModule } from '@angular/common/http';
imports: [
BrowserModule,
HttpClientModule, // import the module
IonicModule.forRoot(MyApp)
]
Solution 2
You are using HttpClient
module instead of HttpClientModule
. So replace the HttpClient
with HttpCLientModule
in both imports as
import { HttpClientModule } from '@angular/common/http';
and
imports: [
BrowserModule,
HttpClientModule,
IonicModule.forRoot(MyApp)
]
Solution 3
Angular 4.3 introduced a new module, HttpClientModule
, which is a complete rewrite of the existing HttpModule
.
import { HttpModule } from '@angular/http';
to:
import { HttpClientModule } from '@angular/common/http';
The second step is to replace every instance of the service Http with the new service HttpClient
.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import { UserModel } from './user.model';
@Injectable()
export class UserService {
constructor(private http: HttpClient) {}
list(): Observable<UserModel> {
return this.http.get('/api/users');
}
}
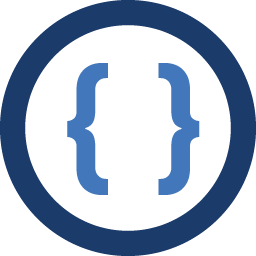
Author by
Admin
Updated on December 15, 2020Comments
-
Admin over 3 years
This is my app.module.ts file
import { BrowserModule } from '@angular/platform-browser'; import { ErrorHandler, NgModule } from '@angular/core'; import { IonicApp, IonicErrorHandler, IonicModule } from 'ionic-angular'; import { SplashScreen } from '@ionic-native/splash-screen'; import { StatusBar } from '@ionic-native/status-bar'; import { HttpClient } from '@angular/common/http'; import { MyApp } from './app.component'; import { HomePage } from '../pages/home/home'; import { DataProvider } from '../providers/data/data'; @NgModule({ declarations: [ MyApp, HomePage, ], imports: [ BrowserModule, HttpClient, IonicModule.forRoot(MyApp) ], bootstrap: [IonicApp], entryComponents: [ MyApp, HomePage ], providers: [ StatusBar, SplashScreen, {provide: ErrorHandler, useClass: IonicErrorHandler}, DataProvider ] }) export class AppModule {}
I’m getting an error like this:
Uncaught Error: Unexpected value 'HttpClient' imported by the module 'AppModule'. Please add a @NgModule annotation. at syntaxError (compiler.js:486) at compiler.js:15240 at Array.forEach (<anonymous>) at CompileMetadataResolver.getNgModuleMetadata (compiler.js:15215) at JitCompiler._loadModules (compiler.js:34413) at JitCompiler._compileModuleAndComponents (compiler.js:34374) at JitCompiler.compileModuleAsync (compiler.js:34268) at CompilerImpl.compileModuleAsync (platform-browser-dynamic.js:239) at PlatformRef.bootstrapModule (core.js:5578) at Object.199 (main.ts:5) syntaxError @ compiler.js:486 (anonymous) @ compiler.js:15240 CompileMetadataResolver.getNgModuleMetadata @ compiler.js:15215 JitCompiler._loadModules @ compiler.js:34413 JitCompiler._compileModuleAndComponents @ compiler.js:34374 JitCompiler.compileModuleAsync @ compiler.js:34268 CompilerImpl.compileModuleAsync @ platform-browser-dynamic.js:239 PlatformRef.bootstrapModule @ core.js:5578 199 @ main.ts:5 __webpack_require__ @ bootstrap c2d88ebb0bb27539f897:54 webpackJsonpCallback @ bootstrap c2d88ebb0bb27539f897:25 (anonymous) @ main.js:1