Unhandled Exception: PlatformException(error, invalid selection start: 24, null, java.lang.IndexOutOfBoundsException: invalid selection start: 24
1,136
The problem is the clearing of the controller.
Instead of
api.addressController.clear();
try:
WidgetsBinding.instance.addPostFrameCallback((_) => api.addressController.clear());
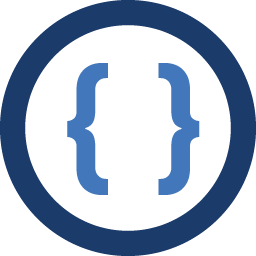
Author by
Admin
Updated on December 29, 2022Comments
-
Admin over 1 year
Whenever I try to clear the TextField by clicking on the IconButton I get an Unhandled Exception warning in the debug console although my TextField is cleared. Also when I try to add a new value to the TextField my previous value is automatically displayed in the TextField. Here's my code for the Search_location UI
import 'package:flutter/material.dart'; import 'package:geoflutterfire/geoflutterfire.dart'; import 'package:geolocator/geolocator.dart'; import 'package:maps_app/models/place.dart'; import 'package:maps_app/services/location_api.dart'; import 'package:provider/provider.dart'; class SearchView extends StatefulWidget { @override _SearchViewState createState() => _SearchViewState(); } class _SearchViewState extends State<SearchView> { final _scrollController = ScrollController(); var latitude, longitude; var con_width = 0.0; var list_height = 0.0; GeoFirePoint point; Geoflutterfire geo = Geoflutterfire(); bool serviceEnabled; LocationPermission permission; @override void initState() { super.initState(); getCurrentLocation(); } getCurrentLocation() async { try { final pos = await Geolocator.getCurrentPosition( desiredAccuracy: LocationAccuracy.high); setState(() { latitude = pos.latitude; longitude = pos.longitude; }); } catch (e) { print(e); } } @override Widget build(BuildContext context) { return Scaffold( body: SearchInjector( child: SafeArea( child: Consumer<LocationApi>( builder: (_, api, child) => SingleChildScrollView( child: Container( padding: const EdgeInsets.all(16), child: Column( children: [ Container( child: TextField( onTap: () { setState(() { list_height = 56; }); }, controller: api.addressController, decoration: InputDecoration( prefixIcon: IconButton( icon: Icon(Icons.my_location), onPressed: () { // getCurrentLocation(); }), suffixIcon: IconButton( icon: Icon(Icons.clear), onPressed: () { setState(() { api.addressController.clear(); con_width = 0.0; list_height = 0.0; }); }), border: OutlineInputBorder( borderSide: BorderSide( color: Colors.red, ), borderRadius: BorderRadius.circular(50.0), ), labelText: 'Search Location', fillColor: Colors.grey[20], filled: true), onChanged: (value) { setState(() { con_width = 300.0; }); api.places.clear(); api.handleSearch(value); }, ), ), AnimatedContainer( duration: Duration(milliseconds: 20), height: list_height, color: Colors.blue[100].withOpacity(.3), width: MediaQuery.of(context).size.width, child: Column( children: List.generate(1, (i) { return ListTile( onTap: () { setState(() { api.addressController.text = 'Your Location'; list_height = 0.0; con_width = 0.0; }); }, title: Row( children: [ Icon(Icons.my_location), SizedBox( width: 5, ), Text( 'Your Location', style: TextStyle( color: Colors.black, fontWeight: FontWeight.bold, ), ), ], ), ); }))), AnimatedContainer( duration: Duration(milliseconds: 20), color: Colors.blue[100].withOpacity(.3), width: MediaQuery.of(context).size.width, height: con_width, child: StreamBuilder<List<Place>>( stream: api.controllerOut, builder: (context, snapshot) { if (snapshot.data == null) { return Center( child: Text('No data address found')); } final data = snapshot.data; return Scrollbar( controller: _scrollController, child: SingleChildScrollView( controller: _scrollController, child: Container( child: Builder(builder: (context) { return Column( children: [ Column( children: List.generate(data.length, (index) { final place = data[index]; return ListTile( leading: Icon(Icons.home), onTap: () { point = geo.point( latitude: place.latitude, longitude: place.longitude); setState(() { api.addressController.text = '${place.name}, ${place.subLocality}, ${place.country}, ${place.postalCode}'; con_width = 0.0; list_height = 0.0; }); }, title: Text( '${place.name}, ${place.subLocality}, ${place.locality}, ${place.postalCode} '), subtitle: Text( '${place.adminArea}, ${place.country}'), ); })), ], ); }), ), ), ); }), ) ], ), ), ), ), ), ), ); } } class SearchInjector extends StatelessWidget { final Widget child; const SearchInjector({Key key, this.child}) : super(key: key); @override Widget build(BuildContext context) { return ChangeNotifierProvider( create: (_) => LocationApi(), child: child, ); } }
This is the Exception I am getting in the debug Console
Restarted application in 1,268ms. E/MethodChannel#flutter/textinput(29061): Failed to handle method call E/MethodChannel#flutter/textinput(29061): java.lang.IndexOutOfBoundsException: invalid selection start: 13 E/MethodChannel#flutter/textinput(29061): at io.flutter.embedding.engine.systemchannels.TextInputChannel$TextEditState.<init>(TextInputChannel.java:724) E/MethodChannel#flutter/textinput(29061): at io.flutter.embedding.engine.systemchannels.TextInputChannel$TextEditState.fromJson(TextInputChannel.java:680) E/MethodChannel#flutter/textinput(29061): at io.flutter.embedding.engine.systemchannels.TextInputChannel$1.onMethodCall(TextInputChannel.java:91) E/MethodChannel#flutter/textinput(29061): at io.flutter.plugin.common.MethodChannel$IncomingMethodCallHandler.onMessage(MethodChannel.java:233) E/MethodChannel#flutter/textinput(29061): at io.flutter.embedding.engine.dart.DartMessenger.handleMessageFromDart(DartMessenger.java:85) E/MethodChannel#flutter/textinput(29061): at io.flutter.embedding.engine.FlutterJNI.handlePlatformMessage(FlutterJNI.java:818) E/MethodChannel#flutter/textinput(29061): at android.os.MessageQueue.nativePollOnce(Native Method) E/MethodChannel#flutter/textinput(29061): at android.os.MessageQueue.next(MessageQueue.java:336) E/MethodChannel#flutter/textinput(29061): at android.os.Looper.loop(Looper.java:174) E/MethodChannel#flutter/textinput(29061): at android.app.ActivityThread.main(ActivityThread.java:7697) E/MethodChannel#flutter/textinput(29061): at java.lang.reflect.Method.invoke(Native Method) E/MethodChannel#flutter/textinput(29061): at com.android.internal.os.RuntimeInit$MethodAndArgsCaller.run(RuntimeInit.java:516) E/MethodChannel#flutter/textinput(29061): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:950) E/flutter (29061): [ERROR:flutter/lib/ui/ui_dart_state.cc(186)] Unhandled Exception: PlatformException(error, invalid selection start: 13, null, java.lang.IndexOutOfBoundsException: invalid selection start: 13 E/flutter (29061): at io.flutter.embedding.engine.systemchannels.TextInputChannel$TextEditState.<init>(TextInputChannel.java:724) E/flutter (29061): at io.flutter.embedding.engine.systemchannels.TextInputChannel$TextEditState.fromJson(TextInputChannel.java:680) E/flutter (29061): at io.flutter.embedding.engine.systemchannels.TextInputChannel$1.onMethodCall(TextInputChannel.java:91) E/flutter (29061): at io.flutter.plugin.common.MethodChannel$IncomingMethodCallHandler.onMessage(MethodChannel.java:233) E/flutter (29061): at io.flutter.embedding.engine.dart.DartMessenger.handleMessageFromDart(DartMessenger.java:85) E/flutter (29061): at io.flutter.embedding.engine.FlutterJNI.handlePlatformMessage(FlutterJNI.java:818) E/flutter (29061): at android.os.MessageQueue.nativePollOnce(Native Method) E/flutter (29061): at android.os.MessageQueue.next(MessageQueue.java:336) E/flutter (29061): at android.os.Looper.loop(Looper.java:174) E/flutter (29061): at android.app.ActivityThread.main(ActivityThread.java:7697) E/flutter (29061): at java.lang.reflect.Method.invoke(Native Method) E/flutter (29061): at com.android.internal.os.RuntimeInit$MethodAndArgsCaller.run(RuntimeInit.java:516) E/flutter (29061): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:950) E/flutter (29061): ) [38;5;244mE/flutter (29061): #0 JSONMethodCodec.decodeEnvelope[39;49m [38;5;244mE/flutter (29061): #1 MethodChannel._invokeMethod[39;49m E/flutter (29061): <asynchronous suspension> E/flutter (29061):