Unhandled Exception: type 'List<dynamic>' is not a subtype of type 'List<Map<String, double>>'
Try:
List<dynamic> t = event;
List<Map<String,double>> newTempList = t[t.length - 1].map((e)=>e as Map<String,double>).toList();
convertedList.add(newTempList);
//Or you can make it shorter by just using this:
List<dynamic> t = event;
convertedList.add(t[t.length - 1].map((e)=>e as Map<String,double>).toList());
Because List<List<Map<String,double>>>
is technically also List<dynamic>
also, but the opposite is not always true. So you have to cast your items as Map,String, double>
first, add those to a list, and then add this newly generated list to your convertedList
.
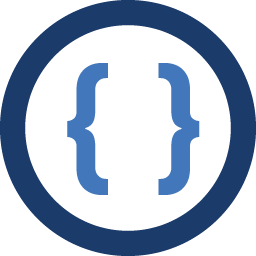
Admin
Updated on November 23, 2022Comments
-
Admin over 1 year
I am very new to using flutter and platform specific code so please forgive me if this is a stupid question. I am using a event channel to return data from android side to flutter. I am returning a List<List<Map<String,double>>> but when it reaches the flutter side, it becomes List dynamic. I want to add the last Map object to another list that I created on the flutter side which has the same type of List<List<Map<String,double>>>.
However, when I tried to add it, it gives an exception, "Unhandled Exception: type 'List' is not a subtype of type 'List<Map<String, double>>'".
This is the list I want to add the Map object to.
List<List<Map<String,double>>> convertedList = [];
This is my adding code. Ignore the print statement.
List<dynamic> t = event; print( "length ${t.length}"); print("type ${t[t.length-1].runtimeType}"); convertedList.add(t[t.length - 1]);
I have tried methods like cast or from but it did not work for me as the same error came out or maybe i used it the wrong way. I really want to know how can i add the Map object to the list. Thanks so much for your help.