Update label's text when pressing a button in Kivy for Python
Solution 1
Well! there was a real need for improvement in your code. (I understand it as you are not experienced.)
Improvement: 1
An application can be built if you return a widget on build(), or if you set self.root.(You shouldn't make all of the gui in build function itself.)
def build(self):
return Hello() #That's what is done here
Improvement: 2
on_release/on_press both are always useful.
self.help_button = Button(text = "Help", size_hint=(.3, .1),pos_hint={'x':.65, 'y':.1},on_press = self.update)
Improvement: 3
As help_button is pressed, update function is called which changes the text of main_label.
def update(self,event):
self.main_label.text = "Changed to change"
Here is you full improved code
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.label import Label
from kivy.uix.floatlayout import FloatLayout
from kivy.clock import Clock
energy = 100
hours = 4
class Hello(FloatLayout):
def __init__(self,**kwargs):
super(Hello,self).__init__(**kwargs)
self.energy_label = Label(text = "Energy = " + str(energy), size_hint=(.1, .15),pos_hint={'x':.05, 'y':.9})
self.time_label = Label(text = "Hours = " + str(hours), size_hint=(.1, .15),pos_hint={'x':.9, 'y':.9})
self.name_label = Label(text = "Game", size_hint=(.1, .15),pos_hint={'x':.45, 'y':.9})
self.main_label = Label(text = "Default_text", size_hint=(1, .55),pos_hint={'x':0, 'y':.35})
#Main Buttons
self.inventory_button = Button(text = "Inventory", size_hint=(.3, .1),pos_hint={'x':.65, 'y':.2})
self.help_button = Button(text = "Help", size_hint=(.3, .1),pos_hint={'x':.65, 'y':.1},on_press = self.update)
self.craft_button = Button(text = "Craft", size_hint=(.3, .1),pos_hint={'x':.05, 'y':.1})
self.food_button = Button(text = "Food", size_hint=(.3, .1),pos_hint={'x':.35, 'y':.2})
self.go_button = Button(text = "Go", size_hint=(.3, .1),pos_hint={'x':.35, 'y':.1})
self.walk_button = Button(text = "Walk", size_hint=(.3, .1),pos_hint={'x':.05, 'y':.2})
self.add_widget(self.energy_label)
self.add_widget(self.main_label)
self.add_widget(self.time_label)
self.add_widget(self.inventory_button)
self.add_widget(self.help_button)
self.add_widget(self.craft_button)
self.add_widget(self.food_button)
self.add_widget(self.go_button)
self.add_widget(self.walk_button)
self.add_widget(self.name_label)
self.current_text = "Default"
def update(self,event):
self.main_label.text = "Changed to change"
class app1(App):
def build(self):
return Hello()
if __name__=="__main__":
app1().run()
Solution 2
Well another way you can go about it is that in Kivy everything at the right of the kivy language properties is pure python. So you can connect your kivy files to the python by passing some tag to your function and then act in python how ever you want.
In the .kv file:
Button:
on_press: root.label_change('btn1')
In the Python file:
Class MyButton(Button):
def label_change(self, event):
self.event = event
if self.event == "btn1":
label.text = "something"
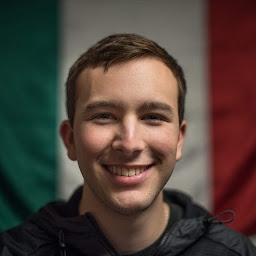
frg100
Updated on June 05, 2022Comments
-
frg100 almost 2 years
Here is my code: I want to make a game where the main_label changes text when you press a button but I've looked everywhere for a week and still don't understand how to do it. I looked on Kivy's website but I don't understand. As you can see I'm new to kivy and not very experienced
from kivy.app import App from kivy.uix.button import Button from kivy.uix.label import Label from kivy.uix.floatlayout import FloatLayout from kivy.clock import Clock energy = 100 hours = 4 class app1(App): def build(self): self.f = FloatLayout() #Labels self.energy_label = Label(text = "Energy = " + str(energy), size_hint=(.1, .15),pos_hint={'x':.05, 'y':.9}) self.time_label = Label(text = "Hours = " + str(hours), size_hint=(.1, .15),pos_hint={'x':.9, 'y':.9}) self.name_label = Label(text = "Game", size_hint=(.1, .15),pos_hint={'x':.45, 'y':.9}) self.main_label = Label(text = "Default_text", size_hint=(1, .55),pos_hint={'x':0, 'y':.35}) #Main Buttons self.inventory_button = Button(text = "Inventory", size_hint=(.3, .1),pos_hint={'x':.65, 'y':.2}) self.help_button = Button(text = "Help", size_hint=(.3, .1),pos_hint={'x':.65, 'y':.1}) self.craft_button = Button(text = "Craft", size_hint=(.3, .1),pos_hint={'x':.05, 'y':.1}) self.food_button = Button(text = "Food", size_hint=(.3, .1),pos_hint={'x':.35, 'y':.2}) self.go_button = Button(text = "Go", size_hint=(.3, .1),pos_hint={'x':.35, 'y':.1}) self.walk_button = Button(text = "Walk", size_hint=(.3, .1),pos_hint={'x':.05, 'y':.2}) def update(self, *args): self.main_widget.text = str(self.current_text) self.f.add_widget(self.energy_label) self.f.add_widget(self.main_label) self.f.add_widget(self.time_label) self.f.add_widget(self.inventory_button) self.f.add_widget(self.help_button) self.f.add_widget(self.craft_button) self.f.add_widget(self.food_button) self.f.add_widget(self.go_button) self.f.add_widget(self.walk_button) self.f.add_widget(self.name_label) self.current_text = "Default" Clock.schedule_interval(update, 1) return self.f def update_label(input): input = self.current_text help_button.bind(on_press = update_label("success!")) if __name__=="__main__": app1().run()
How can I update my code so that by pressing the help_button, main_label changes its text ?
Thank you for your help.
-
frg100 almost 9 yearsThanks for your help! What does the event do in the update function?
-
frg100 almost 9 yearsAlso, how would I add a function so I can change the text I want main_label to output? (So it can be different for each of my buttons)
-
kiok46 almost 9 yearsIt passes instance of that button, try this
print event.text
in update function. -
kiok46 almost 9 yearsPersonally when I deal with such a situation, I love to use kivy language, using ids is always helpful. but since you asked. you can do it this way add this in update function.
if(event.text == "Help"): self.main_label.text = "Help button to change button" elif(event.text == "Go" ): self.main_label.text = "Go button to change button" else: pass
but this is not a good method as text on the button could change. -
kiok46 almost 9 yearsif you want to know about playing with ids in kivy language, then this link might help. stackoverflow.com/questions/30202801/…