Update query PHP MySQL
Solution 1
You have to have single quotes around any VARCHAR content in your queries. So your update query should be:
mysql_query("UPDATE blogEntry SET content = '$udcontent', title = '$udtitle' WHERE id = $id");
Also, it is bad form to update your database directly with the content from a POST. You should sanitize your incoming data with the mysql_real_escape_string function.
Solution 2
Need to add quote for that need to use dot operator:
mysql_query("UPDATE blogEntry SET content = '".$udcontent."', title = '".$udtitle."' WHERE id = '".$id."'");
Solution 3
Without knowing what the actual error you are getting is I would guess it is missing quotes. try the following:
mysql_query("UPDATE blogEntry SET content = '$udcontent', title = '$udtitle' WHERE id = '$id'")
Solution 4
Here i updated two variables and present date and time
$id = "1";
$title = "phpmyadmin";
$sql= mysql_query("UPDATE table_name SET id ='".$id."', title = '".$title."',now() WHERE id = '".$id."' ");
now() function update current date and time.
note: For update query we have define the particular id otherwise it update whole table defaulty
Solution 5
First, you should define "doesn't work".
Second, I assume that your table field 'content' is varchar/text, so you need to enclose it in quotes. content = '{$content}'
And last but not least: use echo mysql_error()
directly after a query to debug.
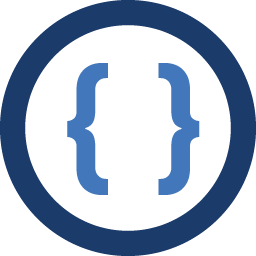
Admin
Updated on October 27, 2020Comments
-
Admin over 3 years
Can anybody help me understand why this update query isn't updating the fields in my database? I have this in my php page to retrieve the current values from the database:
<?php $query = mysql_query ("SELECT * FROM blogEntry WHERE username = 'bobjones' ORDER BY id DESC"); while ($row = mysql_fetch_array ($query)) { $id = $row['id']; $username = $row['username']; $title = $row['title']; $date = $row['date']; $category = $row['category']; $content = $row['content']; ?>
Here i my HTML Form:
<form method="post" action="editblogscript.php"> ID: <input type="text" name="id" value="<?php echo $id; ?>" /><br /> Username: <input type="text" name="username" value="<?php echo $_SESSION['username']; ?>" /><br /> Title: <input type="text" name="udtitle" value="<?php echo $title; ?>"/><br /> Date: <input type="text" name="date" value="<?php echo $date; ?>"/><br /> Message: <textarea name = "udcontent" cols="45" rows="5"><?php echo $content; ?></textarea><br /> <input type= "submit" name = "edit" value="Edit!"> </form>
and here is my 'editblogscript':
<?php mysql_connect ("localhost", "root", ""); mysql_select_db("blogass"); if (isset($_POST['edit'])) { $id = $_POST['id']; $udtitle = $_POST['udtitle']; $udcontent = $_POST['udcontent']; mysql_query("UPDATE blogEntry SET content = $udcontent, title = $udtitle WHERE id = $id"); } header( 'Location: index.php' ) ; ?>
I don't understand why it doesn't work.
-
Rafee over 12 yearsuse
mysql_error()
to find any error with database.. By this way you can solve your issues -
Your Common Sense over 12 yearsechoing doesn't seem convenient method. what if an error occurred while you're away?
-
Your Common Sense over 12 yearsaround any string I believe. say, there are no varchar nor fields at all in the query
SELECT 'Hello World';
but quotes still required. -
Your Common Sense over 12 yearsI'd suggest to use trigger_error(). it will not only follow site-wide error-reporting rules (log or display) but also provide both filename and line number, which info can be priceless in case of logging errors
-
Kerrial Beckett Newham over 7 yearsThis is out of date.
-
mickmackusa about 6 yearsYes, dear modern users, do not use this deprecated and insecure query. You should use
mysqli
orpdo
with prepared statements and placeholders to make the query secure. Never blindly write user-submitted data directly into your query. -
davidethell about 6 yearsYes, for sure this is not the right way to execute a mysql query in php. It answers the original question, but is out of date.