Update TextView every second in Android
Solution 1
You can use following code:
Runnable updater;
void updateTime(final String timeString) {
timer=(TextView) findViewById(R.id.timerText);
final Handler timerHandler = new Handler();
updater = new Runnable() {
@Override
public void run() {
timer.setText(timeString);
timerHandler.postDelayed(updater,1000);
}
};
timerHandler.post(updater);
}
In this line:
timerHandler.post(updater);
time will set for the first time. i.e, updater will execute. After first execution it will be posted after every 1 second time interval. It will update your TextView every one second.
You need to remove it when the activity destroys, else it will leak memory.
@Override
protected void onDestroy() {
super.onDestroy();
timerHandler.removeCallbacks(updater);
}
Hope it will help you.
Solution 2
You should use RxJava library to do so:
Subscription s =
Observable.interval(1, TimeUnit.SECONDS)
.observeOn(AndroidSchedulers.mainThread())
.subscribe(v -> {
// update your ui here
}, e -> {
});
// call when you no longer need an update:
if (s != null && !s.isUnsubscribed()){
s.unsubscribe();
s = null;
}
That's it. Do NOT use .postDelay(), Timer because it is error prone.
Solution 3
You might want to consider using the Chronometer class: https://developer.android.com/reference/android/widget/Chronometer.html
just use timer.start();
on the button click
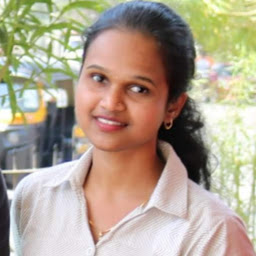
varsha
Updated on June 05, 2022Comments
-
varsha almost 2 years
i want to update my textview every second. on button click i am calling one method,
loopMethod(milli); //suppose milli= 50000 i.e 50 sec.
so my
loopMethod(int m)
is as follows:public void loopMethod(int m){ timer=(TextView) findViewById(R.id.timerText); if(m>=1000){ try { timer.setText(""+m);//timer is a textview System.out.println(m); m=m-1000; Thread.sleep(1000); } catch(InterruptedException ex) { ex.printStackTrace(); } loopMethod(m); } }
so what i am expecting is, my timer
textview
should print the value ofm
every second. but i am getting only console output i.esystem.out.println(m)
... printing value on console working fine... but its not updating my textview at all -
varsha over 7 yearsfacing same problem with above code. :(
-
Pier Giorgio Misley over 7 years@varsha look my "Add" section, we need some more code
-
varsha over 7 yearsabove code gives error: ---android.view.ViewRootImpl$CalledFromWrongThreadException: Only the original thread that created a view hierarchy can touch its views. ------------but after adding comments to timer.setText it perfectly displays output on console with system.out.println
-
varsha over 7 yearsi am just taking milliseconds from user and adding as a parameter to loopMethod. and then after clicking on ok button the timer textview should display the decreasing time after every sec. (Just like countdown)
-
Pier Giorgio Misley over 7 years@varsha check my updated code
-
varsha over 7 yearsThank you so much @Ajith Pandian... thanx a lots.. its working as i wanted :D
-
Ajith Pandian over 7 yearsWelcome. Happy coding.
-
varsha over 7 yearsbut how to stop it? means i want to stop the updating after my timeString>0...to achieve my output i just added timestring-=1000; after timer.setText. and replace the string data type to int. plz help me. i am not familiar with handler
-
Ajith Pandian over 7 yearstimerHandler.removeCallbacks(updater); to stop the handler thread. you can use this whenever you want.
-
varsha over 7 yearsYes, working :D
-
xanexpt almost 7 yearsexactly what i was looking for :) many thanks