Updating existing firewall rule using API
12,663
Solution 1
The code below works for me:
INetFwPolicy2 firewallPolicy = (INetFwPolicy2) Activator.CreateInstance(Type.GetTypeFromProgID("HNetCfg.FwPolicy2"));
var rule = firewallPolicy.Rules.Item("Block Bad IP Addresses"); // Name of your rule here
rule.Name = "Block Block Block"; // Update the rule here. Nothing else needed to persist the changes
Solution 2
In addition to amdmax's answer (sorry I can't add a comment) I found that there is no simple method call to check to see if a rule exists so I came up with this to ensure that a rule is created whether it exists or not:
INetFwPolicy2 firewallPolicy = (INetFwPolicy2)Activator.CreateInstance(
Type.GetTypeFromProgID("HNetCfg.FwPolicy2"));
INetFwRule firewallRule = firewallPolicy.Rules.OfType<INetFwRule>().Where(x => x.Name == RULE_NAME).FirstOrDefault();
if (firewallRule == null)
{
firewallRule = (INetFwRule)Activator.CreateInstance(Type.GetTypeFromProgID("HNetCfg.FWRule"));
firewallRule.Name = RULE_NAME;
firewallPolicy.Rules.Add(firewallRule);
}
Solution 3
I have found this package it is available via nuget WindowsFirewallHelper
PM> install-package WindowsFirewallHelper
Example
var rule = FirewallManager.Instance.Rules.Where(o =>
o.Direction == FirewallDirection.Inbound &&
o.Name.Equals("Allow Remote Desktop")
).FirstOrDefault();
if (rule != null)
{
//Update an existing Rule
rule.RemoteAddresses = new IAddress[]
{
SingleIP.Parse("192.168.184.1"),
SingleIP.Parse("192.168.184.2")
};
return;
}
//Create a new rule
rule = FirewallManager.Instance.CreateApplicationRule(
FirewallManager.Instance.GetProfile().Type,
@"Allow Remote Desktop",
FirewallAction.Allow,
null
);
rule.Direction = FirewallDirection.Inbound;
rule.LocalPorts = new ushort[] { 3389 };
rule.Action = FirewallAction.Allow;
rule.Protocol = FirewallProtocol.TCP;
rule.Scope = FirewallScope.All;
rule.Profiles = FirewallProfiles.Public | FirewallProfiles.Private;
rule.RemoteAddresses = new IAddress[] { SingleIP.Parse("192.168.184.1") };
FirewallManager.Instance.Rules.Add(rule);
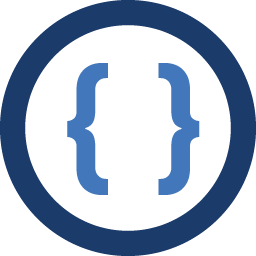
Author by
Admin
Updated on June 06, 2022Comments
-
Admin almost 2 years
I am able to pro grammatically add individual rules to the Windows Firewall (Server 2008 R2), however I am trying to avoid multiple rules per IP address, and would just like to update the existing rule RemoteAddresses. Below is the code I am using to add rules, I am doing my best to research how to update the existing rules Remote Addresses, but with no luck.
Any help is appreciated!
string ip = "x.x.x.x"; INetFwRule2 firewallRule = (INetFwRule2)Activator.CreateInstance(Type.GetTypeFromProgID("HNetCfg.FWRule")); firewallRule.Name = "Block Bad IP Addresses"; firewallRule.Description = "Block Nasty Incoming Connections from IP Address."; firewallRule.Action = NET_FW_ACTION_.NET_FW_ACTION_BLOCK; firewallRule.Direction = NET_FW_RULE_DIRECTION_.NET_FW_RULE_DIR_IN; firewallRule.Enabled = true; firewallRule.InterfaceTypes = "All"; firewallRule.RemoteAddresses = ip; INetFwPolicy2 firewallPolicy = (INetFwPolicy2)Activator.CreateInstance(Type.GetTypeFromProgID("HNetCfg.FwPolicy2")); firewallPolicy.Rules.Add(firewallRule);
-
Arshad about 11 yearsis there any way to unblock the blocked IP, I mean to say update the rule
-
Jeroen K over 10 yearsYou can change rule.RemoteAddresses (comma separated)
-
HABO almost 10 yearsTip for those testing this code: The changes are persisted as described, but may not appear in the Windows Firewall with Advanced Security application until after the display is refreshed.
-
FindOutIslamNow over 6 yearsThat uses
NetFwTypeLib
COM library -
tmm1 about 6 yearsWhat if there are multiple rules with the same name? Windows seems to automatically create two for my app (one for UDP and one for TCP).