updating json object using java
21,751
Solution 1
Parse the JSON and call the particular Key needs to be updated and set the value for the key. Please be more specific.
Create a new JSON Object
private JSONObject Data = new JSONObject;
public Test(){
try {
Data.put("address", username);
}
catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
Solution 2
If you are using the JsonObject class it is immutable
So i believe you have to duplicate the object and change the value like this:
JSONObject object=new JSONObject(jsonstring);
JSONObject childobject=object.getJSONObject("XXXX");
JSONObject modifiedjson=new JSONObject();
modifiedjson.put("type",childobject.get("type"));
modifiedjson.put("value","newvalue"); // Add new value of XXXX here
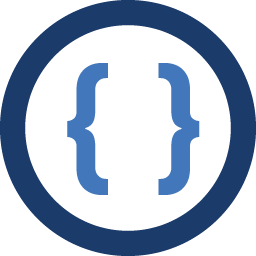
Author by
Admin
Updated on July 07, 2022Comments
-
Admin almost 2 years
Let i've an
JSON
Object like as shown below{"Name":"Manu","Age":"25","Address":""}
Updation
Read the json object and need to update the address field like as given below
{"Name":"Manu","Age":"25","Address":"XXXX"}
can anyone please tell me how to update the Address details in the
JSON
usingjava
My code
JSONObject rec = new JSONObject(data); String name = rec.getString("Name"); String age = rec.getString("Age"); String add = rec.getString("Address");
now how to add some information to the address field
Update 1
String jsonstring="{Name:Manu,Age:25,Address:''}"; JSONObject object=new JSONObject(jsonstring); JSONObject childobject=object.getJSONObject("Address"); JSONObject modifiedjson=new JSONObject(); modifiedjson.put("type",childobject.get("type")); modifiedjson.put("value","newvalue");
Exception
Exception in thread "main" org.json.JSONException: JSONObject["Address"] is not a JSONObject. at org.json.JSONObject.getJSONObject(JSONObject.java:557) at kotouch.Sample.main(Sample.java:59) Java Result: 1
-
Henry over 10 yearsPlease explain what update a Json object means for you. Read the Json, add some information and write it back out or what?
-
mvreijn over 10 yearsYou have to be a little more specific in regard to the steps you need.
-
Admin over 10 yearsafter reading the json object and i need to update the address field.
-
njzk2 over 10 yearsI don't see what is unclear with the error message and all.
-
njzk2 over 10 years(you do see there is no type in you address field, which is a string and not a json object, right ?)
-
saurabheights over 8 yearsThis: "{Name:Manu,Age:25,Address:''}"; is not a json string. This one is json: "{\"Name\":\"Manu\",\"Age\":25,\"Address\":\"\"}"
-
-
Admin over 10 yearsi'm getting exception..............when i tried that ......see my update
-
Rawa over 10 yearsWhat is your exception? Please update the question.
-
Admin over 10 yearssee my update.....................
-
Rawa over 10 yearssimply using docs.oracle.com/javaee/7/api/javax/json/… should cover that case.
-
Admin over 10 yearssir why i'm getting that exception.......;
-
Rawa over 10 yearsYour address field is empty, if it has no value it should be null or non-existing.
-
Admin over 10 yearsi 've put some values in address field still its giving the same exception
-
Rawa over 10 yearsHow do you set the data string? when creating a string with qoutation signs you need to use backslash. I would suggest that you print to make sure that your string really are correct.