Upload image picked from Flutter web to firebase storage and to Firestore
1,038
From the error it seems like the upload expects a File and you are passing Uint8List.
You can try using this to convert the data before uploading it to storage.
File.fromRawPath(Uint8List uint8List);
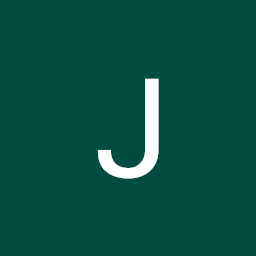
Author by
Tizi Dev
Updated on December 26, 2022Comments
-
Tizi Dev over 1 year
I was able to picked up image from flutter web with the code:
Uint8List uploadedImage; _startFilePicker() async { InputElement uploadInput = FileUploadInputElement(); uploadInput.click(); uploadInput.onChange.listen((e) { // read file content as dataURL final files = uploadInput.files; if (files.length == 1) { final file = files[0]; FileReader reader = FileReader(); reader.onLoadEnd.listen((e) { setState(() { uploadedImage = reader.result; }); }); reader.onError.listen((fileEvent) { setState(() { Text( "Some Error occured while reading the file"); }); }); reader.readAsArrayBuffer(file); } }); } Widget uploadImage() { return Container( width: 530, child: Row( mainAxisAlignment: MainAxisAlignment.center, children: [ uploadedImage == null ? CircleAvatar( radius: 60, backgroundColor: Colors.grey[100], backgroundImage: AssetImage('assets/images/profileavatar.png'), ): CircleAvatar( radius: 65, backgroundImage: AssetImage('assets/images/backgroundslide.gif'), child: CircleAvatar( radius: 60, backgroundImage: MemoryImage(uploadedImage), ), ) , SizedBox( width: 20, ), uploadedImage == null ? RaisedButton( color: Colors.orange, onPressed: () { _startFilePicker(); }, child: Text( 'Aggiungi un immagine profilo', style: TextStyle(color: Colors.white, fontSize: 12), ), ): RaisedButton( color: Colors.orange, onPressed: () { _startFilePicker(); }, child: Text( 'Modifica immagine profilo', style: TextStyle(color: Colors.white, fontSize: 12), ), ), ], ), ); }
in that way I got succesfully the image from Desktop. Now I need to upload this image to storage in flutter and get into Collection in Firestore:
var firebaseUser = FirebaseAuth.instance.currentUser; Future<Uri> uploadImageFile(html.File uploadedImage, {String imageName}) async { fb.StorageReference storageRef = fb.storage().ref('images/$imageName'); fb.UploadTaskSnapshot uploadTaskSnapshot = await storageRef.put(uploadedImage).future; await FirebaseFirestore.instance.collection('ifUser').doc(firebaseUser.uid) .update({"avatarImage": uploadImageFile(uploadedImage),}); Uri imageUri = await uploadTaskSnapshot.ref.getDownloadURL(); return imageUri; }
when I call the function
uploadImageFile(uploadedImage);
I get error:The argument type 'Uint8List' can't be assigned to the parameter type 'File'. Is that a right way?
-
Tizi Dev over 3 yearsCould you give me an example how to code it ?
-
Artemis Georgakopoulou over 3 yearsI haven't reproduced the issue, but the above command is for generating a file from Unit8List. Also I found this Stackoverflow post, which might assist you.