Uploading a file using Spring MVC and Thymeleaf
It seems there is a request method problem.
Your controller's request method is GET
in uploadFile
method but you want to POST
a file in html form.
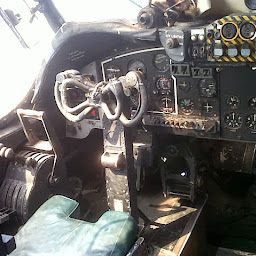
Nicholas Robinson
Updated on July 28, 2022Comments
-
Nicholas Robinson almost 2 years
I am trying to upload a file to the server using a spring controller.
This is my web config:
@Bean public CommonsMultipartResolver multipartConfigElement() { CommonsMultipartResolver commonsMultipartResolver = new CommonsMultipartResolver(); commonsMultipartResolver.setDefaultEncoding("utf-8"); commonsMultipartResolver.setMaxUploadSizePerFile(400000002); return commonsMultipartResolver; } @Override public void addInterceptors(InterceptorRegistry registry) { registry.addInterceptor(securityInterceptor()).addPathPatterns("/**"); } @Bean public TemplateResolver templateResolver() { TemplateResolver resolver = new ServletContextTemplateResolver(); resolver.setPrefix("/WEB-INF/view/"); resolver.setSuffix(".html"); resolver.setTemplateMode("HTML5"); return resolver; } @Bean @Autowired public SpringTemplateEngine templateEngine(TemplateResolver resolver) { SpringTemplateEngine engine = new SpringTemplateEngine(); engine.setTemplateResolver(resolver); return engine; } @Bean @Autowired public ViewResolver viewResolver(SpringTemplateEngine engine) { ThymeleafViewResolver resolver = new ThymeleafViewResolver(); resolver.setTemplateEngine(engine); return resolver; }
And this is my controller:
@RequestMapping(value = "/upload", method = RequestMethod.GET) public String uploadFile(HttpServletRequest request) throws IOException, ServletException { System.out.println("Request: " + request); // REM: Debug Print final String path = request.getParameter("destination"); final Part filePart = request.getPart("file"); System.out.println("Path: " + path); // REM: Debug Print System.out.println("File Part toString: " + filePart); // REM: Debug Print if (filePart != null) { System.out.println("File Part name: " + filePart.getName()); // REM: Debug Print System.out.println("File Part size: " + filePart.getSize()); // REM: Debug Print } else { System.out.println("Parts is null"); // REM: Debug Print } return "file_upload_testing"; }
The class is annotated with
@MultipartConfig
.I am using Thymeleaf on the front end and I have created a simple form to try get the upload working as follows:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="http://www.thymeleaf.org" lang="en"> <head> <title>File Upload</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"/> </head> <body> <form method="POST" action="upload" enctype="multipart/form-data" > <input type="file" name="file" id="file" /> <br/> <input type="text" value="/tmp" name="destination"/><br/> <input type="submit" value="Upload" name="upload" id="upload" /> </form> </body> </html>
All my other controllers are working, I just cannot get the
MultipartFile
into the controller.I have tried using the
MultipartFile
as a parameter into the my controller and I have also tried casting theHttpServletRequest
to a Multipart request. None of this made any difference.When I make the request to the controller with a text file this is the output:
Request: org.apache.catalina.connector.RequestFacade@48d0006b Path: null File Part toString(): null Parts is null
Please help my figure out why the file is not coming through with the request?
[UPDATE] I have tried changing the request mapping to
@RequestMapping(value = "/upload", method = RequestMethod.POST)
but still get the same output.I have now also tried:
Controller:
@RequestMapping(value = "/upload", method = RequestMethod.POST) public String uploadFile(MultipartFile file) throws IOException, ServletException { System.out.println("File: " + file); // REM: Debug Print return "file_upload_testing"; }
Web config:
@Bean public MultipartResolver mulitpartResolver() { CommonsMultipartResolver commonsMultipartResolver = new CommonsMultipartResolver(); commonsMultipartResolver.setDefaultEncoding("utf-8"); commonsMultipartResolver.setMaxUploadSizePerFile(400000002); return commonsMultipartResolver; }
With this I get:
java.lang.IllegalArgumentException: Expected MultipartHttpServletRequest: is a MultipartResolver configured?