Uploading file using POST request in Flutter
Solution 1
The correct way is to use a MultipartRequest:
var uri = Uri.parse(url);
var request = new MultipartRequest("POST", uri);
var multipartFile = await MultipartFile.fromPath("package", videoPath);
request.files.add(multipartFile);
StreamedResponse response = await request.send();
response.stream.transform(utf8.decoder).listen((value) {
print(value);
});
Solution 2
You can use the Dio package. It supports large files and works fine with me.
sendFile(String kMainUrl, XFile file) async {
String filePath = file.path;
String fileName = 'any name';
try {
FormData formData = FormData.fromMap({
"file":
await MultipartFile.fromFile(filePath, filename:fileName),
});
Response response =
await Dio().post(kMainUrl, data: formData);
print("File upload response: $response");
print(response.data['message']);
} catch (e) {
print("Exception Caught: $e");
}
}
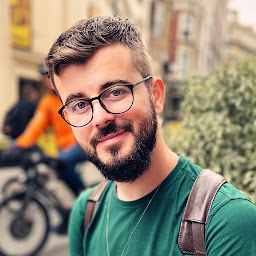
Razvan Cristian Lung
I'm a forward thinking developer offering more than two years of experience building, integrating, testing and supporting Flutter and Android applications. I like projects that put efficiency and user experience first. Though sometimes this might be tough, I like to be presented to difficult situations. I've been learning Android as a hobby, but it soon became one of my favorite things to do. I like to make full use of the Firebase SDK. And since Flutter came out I just fell in love with it. It is so versatile and performant. Take note: This is the future. (Fuchsia I'm waiting for you)
Updated on December 03, 2022Comments
-
Razvan Cristian Lung over 1 year
I'm trying to upload a video file to my server using a post request.
var file = new File(videoPath); var uri = Uri.parse(tokenizedUri); HttpClientRequest request = await new HttpClient().postUrl(uri); await request.addStream(file.openRead()); var response = await request.close(); response.transform(utf8.decoder).forEach((string) { print(string); // handle data });
But the server doesn't get it. Why?