Use Axios to get and save an Excel file
Solution 1
Try removing responseType
from the headers and add it directly into your options object that you pass into axios:
axios({
method:'GET',
url: '[your_url_to_get_file]',
responseType: 'blob',
headers: { your headers }
})
On top of that, I would try using application/vnd.openxmlformats-officedocument.spreadsheetml.sheet
as your content-type, instead of octect-stream
. I've never tried octect-stream
but the one I mentioned has always worked for me with .xlsx files.
For reference about MIME types see here.
Solution 2
const axios = require('axios');
const fs = require('fs');
const getXLS = () => {
return axios.request({
responseType: 'arraybuffer',
url: 'https://drive.google.com/ft7P9FAQ/view?usp=sharing',
method: 'get',
headers: {
'Content-Type': 'blob',
},
}).then((result) => {
const outputFilename = 'xyzzzz.xls';
fs.writeFileSync(outputFilename, result.data);
return outputFilename;
});
}
getXLS();
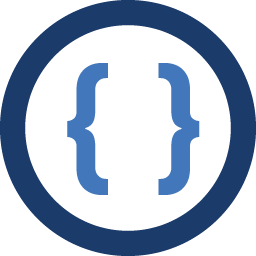
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I am using react and axios to do a POST to a php service that will return a .xlsx file. The .xlsx file is created properly on the server, but during transit back it gets corrupted, through the Chrome inspector the data seems to be getting converted to a string and many characters are getting changed as a result. Is there a way to do this? Things I have tried:
Headers on request
'Accept': 'application/octet-stream', 'responseType': 'blob',
With the response object
fileDownload(new Blob([response.data]), 'report.xlsx');
Alternatively with the response object
const url = window.URL.createObjectURL(new Blob([response.data])); const link = document.createElement('a'); link.href = url; link.setAttribute('download', 'file.xlsx'); link.click();
No matter what I try it seems to be getting corrupted. On the server side I have the following PHP code:
$response = new Stream(); $response->setStream(fopen($tempFilePath, 'r')); $response->setStatusCode(200); $response->setStreamName($tempFilePath); $responseHeaders = new Headers(); $responseHeaders->addHeaders(array( 'Content-Disposition' => 'attachment; filename='report.xlsx', 'Content-Type' => 'application/octet-stream', 'Content-Length' => filesize($tempFilePath), 'Expires' => '@0', 'Cache-Control' => 'must-revalidate', 'Pragma' => 'public' )); $response->setHeaders($responseHeaders); return $response;