Use Enum.values in with generic class in dart
You can't do this. First of all Enum
class doesn't contain values
list. The second reason is values
in enum is a static field and you can't call static methods or fields on the generic type even you cast them to the specific one.
If you want to create T? getEnum<T>(String key)
you can achieve this but in a bad way. So it is just an example:
T? getEnum<T>(String key) {
if(T == GenderType) {
//needs some additional checks if _pref is null or getInt(key) returned null
return GenderType.values[_pref?.getInt(key)] as T;
}
//if(T == anotherType) {}
//and so on
throw Exception("Unhandled type: $T");
}
But much better to use a separate method for each type.
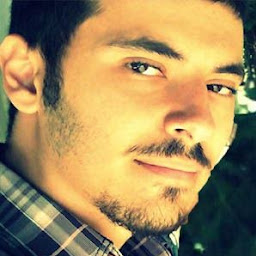
Umut ADALI
I have been writing code in different languages for a long time. I basically go through android and I love ruby very much.
Updated on January 02, 2023Comments
-
Umut ADALI over 1 year
This question was asked nine years ago for javascript but I couldn't find the answer for the dart. I try to achieve json serialization with enum. There are some solutions with libraries but I want to answer dart logic.
enum GenderType{ Male, Female, NonBinary }
T? getEnum<T>(String key) { return (T as Enum).values[_pref?.getInt(key)]; }
I want to write like this. Although I can call GenderType.values, I cannot call them as T.values.
-
Umut ADALI about 2 yearsIt's not generic type. I don't want to use GenderType in getEnum function. Because there are lots of different enum class like GenderType and this isn't reusable like this code block