Use Navigator.push (MaterialPageRoute) instead of AlertDialog
1,661
Create a StatefulWidget
subclass say MyForm
.
class MyForm extends StatefulWidget {
@override
_MyFormState createState() => _MyFormState();
}
class _MyFormState extends State<MyForm> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text("My form")),
body: Form(
key: formKey,
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Padding(
padding: EdgeInsets.all(8.0),
child: TextFormField(
initialValue: '',
onSaved: (val) => board.subject = val,
validator: (val) => val == "" ? val : null,
),
),
Padding(
padding: EdgeInsets.all(8.0),
child: RaisedButton(
color: Colors.indigo,
child: Text(
'Post',
style: TextStyle(color: Colors.white),
),
onPressed: () {
handleSubmit();
Navigator.of(context).pop();
},
),
)
],
),
),
);
}
}
And use it like this in your onPressed
method.
onPressed: () {
Navigator.push(context, MaterialPagerRoute(builder: (context) => MyForm()));
}
So, when the button is clicked, you will be navigated to the new page which is your form currently.
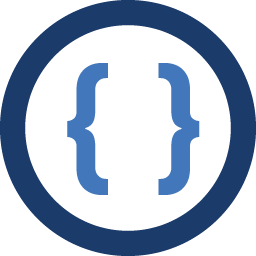
Author by
Admin
Updated on December 12, 2022Comments
-
Admin over 1 year
I would like to use Navigator.push (MaterialPageRoute) instead of AlertDialog as now I think its better for my user to have a full page to post content rather than a dialog box, how would I go about editing my code to do this? Thanks in advance
appBar: AppBar( centerTitle: true, title: Text('hehe', style: TextStyle(fontWeight: FontWeight.bold, fontSize: 25.0),), actions: <Widget>[ Padding( padding: const EdgeInsets.only(right: 10.0), child: IconButton(icon: Icon(Icons.comment), onPressed: () { showDialog(context: context, builder: (BuildContext context){ return AlertDialog( shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10.0)), content: Form(key: formKey, child: Column( mainAxisSize: MainAxisSize.min, children: <Widget>[ Padding( padding: EdgeInsets.all(8.0), child: TextFormField( initialValue: '', onSaved: (val) => board.subject = val, validator: (val) => val == "" ? val: null, ), ), Padding( padding: EdgeInsets.all(8.0), child: RaisedButton( color: Colors.indigo, child: Text( 'Post', style: TextStyle(color: Colors.white),), onPressed: () { handleSubmit(); Navigator.of(context).pop(); }, ), ) ], ), ), ); }, ); } ), ), ], ),
-
Mariano Zorrilla almost 5 yearsCreate a new StatefulWidget with the content of your AlertDialog. That way you could do the Navigator to move to that page.
-
Admin almost 5 yearsi'm lost, its currently in a stateful widget
-
Mariano Zorrilla almost 5 yearsyes, but if you want to use Navigator.push you need to move to a new StatefulWidget... that's the way to can have a full screen modal.
-