Using a variable inside a Chef recipe
Solution 1
The source of the problem here is that you are setting the variable fqdn inside the scope of a ruby_block and are attempting to make reference to that variable at the compilation phase. The ruby_block resources allows the ability to run ruby code during the convergence phase.
Given that you appear to be using the fqdn to setup the resource set, it looks as though you can remove the ruby block from around the ruby code. e.g.
fqdn = // logic to get fqdn
file '/tmp/file' do
content "fqdn=#{fqdn}"
end
Solution 2
I found this in the Chef docs. I ran into a similar issue. I am going to try the node.run_state
. This information is found at the bottom of this page https://docs.chef.io/recipes.html
Use
node.run_state
to stash transient data during a chef-client run. This data may be passed between resources, and then evaluated during the execution phase.run_state
is an empty Hash that is always discarded at the end of the chef-client run.For example, the following recipe will install the Apache web server, randomly choose PHP or Perl as the scripting language, and then install that scripting language:
package "httpd" do action :install end ruby_block "randomly_choose_language" do block do if Random.rand > 0.5 node.run_state['scripting_language'] = 'php' else node.run_state['scripting_language'] = 'perl' end end end package "scripting_language" do package_name lazy { node.run_state['scripting_language'] } action :install end
Solution 3
Please follow this link http://lists.opscode.com/sympa/arc/chef/2015-03/msg00266.html you can use node.run_state[:variables] for parsing one variable to another recipe
Here is my code :: file.rb
node.run_state[:script_1] = "foo" include_recipe 'provision::copy'
and in other copy.rb file put following code ::
copy.rb
filename = node.run_state[:script_1] puts "Name is #{filename}"
Solution 4
I used node.run_state['variable']
for the same purpose and was successfully able to do it. Please find basic sample code below.
ruby_block "resource_name" do
block do
node.run_state['port_value'] = 1432
end
end
ruby_block "resource_name2" do
block do
num = node.run_state['variable']
end
end
I hope it will help.
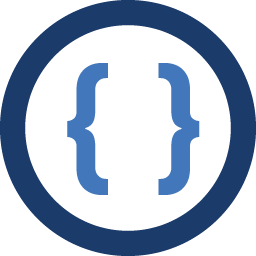
Admin
Updated on June 16, 2020Comments
-
Admin almost 4 years
I'm using chef-cookbook-hostname cookbook to setup node's hostname. I don't want my hostname to be hard coded in the attribute file (default['set_fqdn']).
Instead the hostname will be read from a VM definition XML file. I come up with the following default recipe but apparently the variable fqdn is not given value. Is there any idea why this happens or any better to achieve my task?
ruby_block "Find-VM-Hostname" do block do require 'rexml/document' require 'net/http' url = 'http://chef-workstation/services.xml' file = Net::HTTP.get_response(URI.parse(url)).body doc = REXML::Document.new(file) REXML::XPath.each(doc, "service_parameters/parameter") do |element| if element.attributes["name"].include?"Hostname" fqdn = element.attributes["value"] #this statement does not give value to fqdn end end end action :nothing end if fqdn fqdn = fqdn.sub('*', node.name) fqdn =~ /^([^.]+)/ hostname = Regexp.last_match[1] case node['platform'] when 'freebsd' directory '/etc/rc.conf.d' do mode '0755' end file '/etc/rc.conf.d/hostname' do content "hostname=#{fqdn}\n" mode '0644' notifies :reload, 'ohai[reload]' end else file '/etc/hostname' do content "#{hostname}\n" mode '0644' notifies :reload, 'ohai[reload]', :immediately end end