Using async without await?
Solution 1
If your Logger.LogInfo
is already async this is enough:
public void PushCallAsync(CallNotificationInfo callNotificationInfo)
{
Logger.LogInfo("Pushing new call {0} with {1} id".Fill(callNotificationInfo.CallerId,
}
If it is not just start it async without waiting for it
public void PushCallAsync(CallNotificationInfo callNotificationInfo)
{
Task.Run(() => Logger.LogInfo("Pushing new call {0} with {1} id".Fill(callNotificationInfo.CallerId));
}
Solution 2
You still are misunderstanding async
. The async
keyword does not mean "run on another thread".
To push some code onto another thread, you need to do it explicitly, e.g., Task.Run
:
await Task.Run(() => Logger.LogInfo("Pushing new call {0} with {1} id".Fill(callNotificationInfo.CallerId));
I have an async
/await
intro post that you may find helpful.
Solution 3
You're misunderstanding async
.
It actually just tells the Compiler to propagate the inversion of control flow it does in the background for you. So that the whole method stack is marked as async.
What you actually want to do depends on your problem. (Let's consider your call Logger.LogInfo(..)
is an async
method as it does eventually call File.WriteAsync() or so.
- If you calling function is a void event handler, you're good. The async call will happen to some degree (namely File.WriteAsync) in the background. You do not expect any result in your control flow. That is fire and forget.
- If however you want to do anything with the result or if you want to continue only then, when
Logger.LogInfo(..)
is done, you have to do precautions. This is the case when your method is somehow in the middle of the call-stack. ThenLogger.LogInfo(..)
will usually return aTask
and that you can wait on. But beware of calling task.Wait() because it will dead lock your GUI-Thread. Instead use await or return the Task (then you can omit async):
public void PushCallAsync(CallNotificationInfo callNotificationInfo)
{
return Logger.LogInfo("Pushing new call {0} with {1} id".Fill(callNotificationInfo.CallerId);
}
or
public async void PushCallAsync(CallNotificationInfo callNotificationInfo)
{
await Logger.LogInfo("Pushing new call {0} with {1} id".Fill(callNotificationInfo.CallerId);
}
Solution 4
If Logger.LogInfo is a synchronous method, the whole call will be synchronous anyway. If all you want to do is to execute the code in a separate thread, async is not the tool for the job. Try with thread pool instead:
ThreadPool.QueueUserWorkItem( foo => PushCallAsync(callNotificationInfo) );
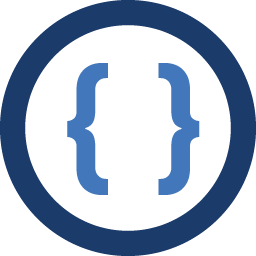
Admin
Updated on July 02, 2021Comments
-
Admin almost 3 years
Consider Using async without await.
think that maybe you misunderstand what async does. The warning is exactly right: if you mark your method async but don't use await anywhere, then your method won't be asynchronous. If you call it, all the code inside the method will execute synchronously.
I want write a method that should run async but don't need use await.for example when use a thread
public async Task PushCallAsync(CallNotificationInfo callNotificationInfo) { Logger.LogInfo("Pushing new call {0} with {1} id".Fill(callNotificationInfo.CallerId, }
I want call
PushCallAsync
and run async and don't want use await.Can I use async without await in C#?