Using command line argument range in bash for loop prints brackets containing the arguments
Solution 1
How about:
for i in $(eval echo {$1..$2}); do echo $i; done
Solution 2
You can slice the input using ${@:3}
or ${@:3:8}
and then loop over it
For eg., to print arguments starting from 3
for i in ${@:3} ; do echo $i; done
or to print 8 arguments starting from 3 (so, arguments 3 through 10)
for i in ${@:3:8} ; do echo $i; done
Solution 3
Use the $@ variable?
for i in $@
do
echo $i
done
If you just want to use 1st and 2nd argument , just
for i in $1 $2
If your $1 and $2 are integers and you want to create a range, use the C for loop syntax (bash)
for ((i=$1;i<=$2;i++))
do
...
done
Solution 4
I had a similar problem. I think the issue is with dereferencing $1 within the braces '{}'. The following alternative worked for me ..
#!/bin/bash
for ((i=$1;i<=$2;i++))
do
...
done
Hope that helps.
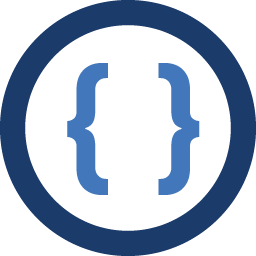
Admin
Updated on July 17, 2021Comments
-
Admin almost 3 years
It's probably a lame question. But I am getting 3 arguments from command line [ bash script ]. Then I am trying to use these in a for loop.
for i in {$1..$2} do action1 done
This doesn't seem to work though and if
$1
is"0"
and$2
is2
it prints{0..2}' and calls
action1` only once. I referred to various examples and this appears to be the correct usage. Can someone please tell me what needs to be fixed here?Thanks in advance.
-
l0b0 over 12 yearsYou might want to only include the second part of the answer - It's better than using
eval
and fits the question. -
nhed over 10 years+1 IMO This is cleaner than having to
eval
as in the accepted answer, but the accepted answer is more specific to the OP -
Yogarine over 2 yearsI would recommend against using eval and consider splicing up the arguments as suggested in Vijayender's answer.