Using gluPerspective()
gluPerspective(45, 1.333, 0, 100);
Never set zNear to zero. It messes up the math:
Because
r = zFar / zNear
approaches infinity aszNear
approaches 0,zNear
must never be set to 0.
Choose some small positive number like 0.01
or 12
.
EDIT: Also, put your camera somewhere such that it can see your geometry:
int main( int argc, char **argv)
{
init_perspective();
glTranslatef(0,0,-5);
glColor4f(1.0,1.0,1.0,1.0);
glBegin(GL_QUADS);
glVertex2f(-1,-1);
glVertex2f( 1,-1);
glVertex2f( 1, 1);
glVertex2f(-1, 1);
glEnd();
SDL_GL_SwapBuffers();
SDL_Delay(1000);
SDL_Quit();
return 0;
}
By default it's looking down the -Z axis from position (0,0,0).
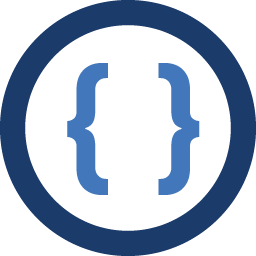
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm learning OpenGL; I managed to make my first 2D program but I am stuck on using gluPerspective.
I'm trying a little test to try to grasp how I can go about making a 3D project but I can't seem to figure out what I am missing and what is needed to make this small test work:
void init_perspective() { SDL_Init(SDL_INIT_EVERYTHING); SDL_SetVideoMode(640, 480, 32, SDL_OPENGL); SDL_WM_SetCaption( "OpenGL Test", NULL ); glClearColor( 0, 0, 0, 0 ); glMatrixMode( GL_PROJECTION ); glLoadIdentity(); gluPerspective(45, 1.333, 0, 100); glMatrixMode( GL_MODELVIEW ); glLoadIdentity(); glClear( GL_COLOR_BUFFER_BIT ); } int main( int argc, char **argv) { init_perspective(); glTranslatef( 200, 200, 50 ); glColor4f(1.0,1.0,1.0,1.0); glBegin(GL_QUADS); glVertex3f(0, 0 ,0); glVertex3f(50,0 ,10); glVertex3f(50,50,30); glVertex3f(0, 50,0); glEnd(); glLoadIdentity(); SDL_GL_SwapBuffers(); SDL_Delay(1000); SDL_Quit(); return 0; }
If anybody could tell me what function calls I could use and are missing in this test code so I could have a play around and learn how 3D OpenGL works I would be grateful.