Using google drive to stream video into html5 player
Solution 1
Short answer: Nope
Longer answer: Oh boy. I spent quite a long time trying to figure this one out myself! I'm working on a project called DriveStream, that aims to use purely JavaScript and Apps Script to make an organised video library of a Drive account.
The project itself works fine, but implementing video streaming did not go anywhere near as planned. I tried a few different methods. One similar to yours and the other involving getting the downloadUrl
property of a file and parsing the html of the download prompt page to give me a direct link to the file. I can initiate a download of that file, and that can be seen in the network requests, but there is no way to get it to stream into a video container.
The reason that doesn't work is due to the limitations of AJAX. It can return the data from a video, but there seems to be no way to funnel that data into a video container.
In the end, I've had to compensate by having the 'Play' button of each video only link to the preview video.
https://drive.google.com/file/d/fileId/preview
It's not a bad workaround, as it can play up to 1080p encodes of anything you upload.
Solution 2
// This works...
<video src="https://www.googleapis.com/drive/v3/files/fileID?alt=media&key=apiKey">
// But, even better if you have access to a worker or can otherwise output the file through a script (and modify resonse headers)
async function getFile(request) {
let response = await fetch('https://www.googleapis.com/drive/v3/files/fileID?alt=media&key=apiKey', request)
response = new Response(response.body, response)
response.headers.set('Accept-Ranges', 'bytes') // Allow seeking, not necessarily enabled by default
response.headers.set('Content-Disposition', 'inline') // Disable Google's attachment (download) behaviour, display directly in browser or stick into video src instead
return response
}
addEventListener('fetch', event => {
event.respondWith(getFile(event.request))
})
Solution 3
It's a long answer I will try to shorten it up..
- Upload your file to the drive and using the share option make it public.
- Then click on the preview option, and it will open a new tab.
- Look for the vertical 3 dots (top right corner) leading to a sub-menu and select open in a new window.
- As soon as you get to the new window click on the vertical 3 dots (top right corner) and this time you will see an 'Embed item' option click it.
- You will get the iframe code now include it your webpage or website.
Just give it a try once!!! I hope this helps thank you!!
Solution 4
This should work:
<video>
<source src="https://drive.google.com/uc?export=download&id=file_ID" type='video/mp4'>
</video>
Replace "file_ID" in the link with your video ID. (to find it - right click on the Google Drive video, get shareable link. The file should be shared in order to access the file.)
It plays the source video. If you would like to change quality or to use unsupported codecs it's not easy to get google drive stream/converted files as said in previous answer. (Ajax will not let you get the link because of CORS and PHP is server side and the link contains an IP address so will not be accessible directly from client side.)
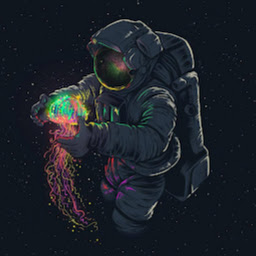
Tim Untersberger
Updated on June 05, 2022Comments
-
Tim Untersberger almost 2 years
I tried to use google drive as a place to host videos, but whenever I try to use the video as a source, google never returns anything. (Not even a http response)
Example video player
<video> <source src="https://www.googleapis.com/drive/v3/files/<file_id>/?alt=media&key=<api_key>" type="video/mp4" /> </video>
If I enter the url in the browser it successfully prompts me for permission to download.
Does anybody have any idea what to do?
-
SeaBass over 5 yearsThis doesn't work anymore right? Any other solution?
-
SeaBass over 5 yearsDoes this still work? The example link you posted works in the browser (when adding my video ID of course), but it seems like it still opens in it's own player and when I look at the link that google is using it's a mile long and include info about expiring and things. I can't get your link ending with preview to play right in my html5 player. Ideas? Thanks!
-
stewartmcgown over 5 yearsOnly works for files small enough for google to scan. So less than 1GB. Useless for my project, but good enough for smaller ones!
-
pejey over 5 yearsWhen you exceed the file size limit for the virus scan you need to get the link from the "Download anyway" button. There is only one more parameter needed - &confirm=something. Here is one way how to get it - marstranslation.com/blog/…
-
SeaBass over 5 years@pejey Thank you! My test file is 231MB and it still can't play. I get the virus message. 1GB would be fine for me, but it seems like the limit is lower which will not work. Anyone know what the limit is? Also it seems like the confirm ID is changing so that doesn't seem reliable either and I'd need it to be accessible automatically, not by using the console and things unfortunately. I have less tech-savy people who will use my app and I want them to just have to paste a shared link. Please let me know if you have any ideas. Thanks!
-
pejey over 5 yearsAt this moment it is 100MB as stated here - support.google.com/a/answer/172541?hl=en
-
pejey over 5 yearsI haven't needed larger files but this could probably help to get the confirmation ID stackoverflow.com/a/49523486/9583275 . Just in case, when the video is not playable I would put there an iframe with drive.google.com/file/d/file_ID/preview so the user still could play it (unfortunately without your controls or filters)
-
SeaBass over 5 yearsThank you, that could work! Except for downloading the page to snatch the confirm code, do you think there is a way to calculate it, or is that a google secret?
-
alsuren over 4 yearsI think I have worked out a scheme for making google do the heavy-lifting of serving the videos, but it requires a bunch of hackery (probably easier to just pay for 15GB of CDN hosting): 100MB limit: use ffmpeg's HLS mode to split up your video into a bunch of segment_*.ts files and a .m3u8 playlist file, and use hls.js to play it. CORS: serve the .m3u8 playlist file from a smart server (when a request is made, map each of the filenames into file ids, and then use requests.get(url, allow_redirects=False).headers['location'] to get the real url for each segment).