Using jQuery, Restricting File Size Before Uploading
Solution 1
This is a copy from my answers in a very similar question: How to check file input size with jQuery?
You actually don't have access to the filesystem (for example reading and writing local files). However, due to the HTML5 File API specification, there are some file properties that you do have access to, and the file size is one of them.
For this HTML:
<input type="file" id="myFile" />
try the following:
//binds to onchange event of your input field
$('#myFile').bind('change', function() {
//this.files[0].size gets the size of your file.
alert(this.files[0].size);
});
As it is a part of the HTML5 specification, it will only work for modern browsers (v10 required for IE) and I added here more details and links about other file information you should know: http://felipe.sabino.me/javascript/2012/01/30/javascipt-checking-the-file-size/
Old browsers support
Be aware that old browsers will return a null
value for the previous this.files
call, so accessing this.files[0]
will raise an exception and you should check for File API support before using it
Solution 2
I don't think it's possible unless you use a flash, activex or java uploader.
For security reasons ajax / javascript isn't allowed to access the file stream or file properties before or during upload.
Solution 3
I tried it this way and I am getting the results in IE*, and Mozilla 3.6.16, didnt check in older versions.
<img id="myImage" src="" style="display:none;"><br>
<button onclick="findSize();">Image Size</button>
<input type="file" id="loadfile" />
<input type="button" value="find size" onclick="findSize()" />
<script type="text/javascript">
function findSize() {
if ( $.browser.msie ) {
var a = document.getElementById('loadfile').value;
$('#myImage').attr('src',a);
var imgbytes = document.getElementById('myImage').size;
var imgkbytes = Math.round(parseInt(imgbytes)/1024);
alert(imgkbytes+' KB');
}else {
var fileInput = $("#loadfile")[0];
var imgbytes = fileInput.files[0].fileSize; // Size returned in bytes.
var imgkbytes = Math.round(parseInt(imgbytes)/1024);
alert(imgkbytes+' KB');
}
}
</script>
Add Jquery library also.
Solution 4
$(".jq_fileUploader").change(function () {
var fileSize = this.files[0];
var sizeInMb = fileSize.size/1024;
var sizeLimit= 1024*10;
if (sizeInMb > sizeLimit) {
}
else {
}
});
Solution 5
I encountered the same issue. You have to use ActiveX or Flash (or Java). The good thing is that it doesn't have to be invasive. I have a simple ActiveX method that will return the size of the to-be-uploaded file.
If you go with Flash, you can even do some fancy js/css to cusomize the uploading experience--only using Flash (as a 1x1 "movie") to access it's file uploading features.
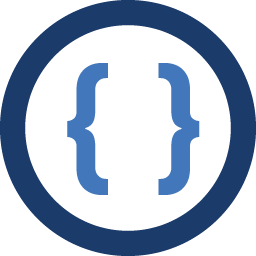
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
On PHP, they have a way to restrict file size AFTER uploading, but not BEFORE uploading. I use the Malsup jQuery Form Plugin for my form posting, and it supports image file posting.
I was wondering if perhaps there's a restriction where I can set how many bytes can pass through that AJAX stream up to the server? That could permit me to check that file size and return an error if the file is too big.
By doing this on the client side, it blocks those newbies who take a 10MB photo shot from their Pentax and try to upload that.
-
Tom almost 15 yearsThis seems like the way to go and I'm pretty sure that Flickr uses a Flash control to provide some feedback on what your uploading, if it's too big and it's progress.
-
Doug Molineux about 13 yearsFirst of all, thank you for the code. Secondly, I have this code located here: jsfiddle.net/6ZQkj/2 It's not working in Chrome, Firefox or Safari on a Mac, any insight welcome
-
Purefan over 12 yearsWe use SWFUpload, although not in active development it has proven quite handy and easy to integrate
-
Felipe Sabino about 12 yearsThis was actually true by the time you wrote this answer, but we do now have a File API in the HTML5 new specification :) stackoverflow.com/a/9106313/429521
-
Felipe Sabino almost 11 years@ShawnEary it's not a trick, its is just the HTML5 File API implementation, which IE10 indeed supports caniuse.com/#feat=fileapi :)
-
Ilya Luzyanin over 9 yearsThe question is rather old, you have plenty of time to make a good descriptive answer, i.e. add some explanations to your code please.
-
Stiger about 9 yearsI think it not works with IE 9, file object doesn't have size property.