Using mapper & fileset to copy files into a different subdirectory?
You could use a regexp
mapper:
<copy todir="${dest.dir}">
<fileset dir="${src.dir}" casesensitive="yes">
<include name="**/*.pdf"/>
</fileset>
<mapper type="regexp" from="^(.*)/(.*\.pdf)" to="\1/x/\2" />
</copy>
I've used hard-coded file.separators to shorten. Basically, you split the path to the input file (from) into directory and filename (capture \1
and \2
) and then insert the \x
extra element between them (to).
I'm not clear on your example - it looks like you want to match 'bar.pdf' and rename it to 'foo.pdf', as well as changing the directory. If you need to do that, you might consider chaining a couple of simpler regexp mappers, rather than trying to cook up one complex one:
<copy todir="${dest.dir}">
<fileset dir="${src.dir}" casesensitive="yes">
<include name="**/*.pdf"/>
</fileset>
<chainedmapper>
<mapper type="regexp" from="^(.*)/(.*\.pdf)" to="\1/x/\2" />
<mapper type="regexp" from="^(.*)/(.*\.pdf)" to="\1/foo.pdf" />
</chainedmapper>
</copy>
When using a glob
mapper, you need to specify one wildcard *
in the from field:
Both to and from are required and define patterns that may contain at most one *. For each source file that matches the from pattern, a target file name will be constructed from the to pattern by substituting the * in the to pattern with the text that matches the * in the from pattern. Source file names that don't match the from pattern will be ignored.
So something like this might work:
<mapper type="glob" from="*/foo.pdf" to="*/x/foo.pdf" />
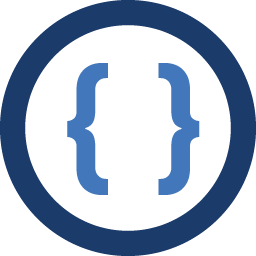
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to create an Ant target that copies files in a directory to a destination directory with the same folder structure, plus one more subfolder appended.
For example, the source is:
a/b/c/foo.pdf d/e/f/bar.pdf
I want the destination to be:
a/b/c/x/foo.pdf d/e/f/x/bar.pdf
Here is my target so far, but it doesn't appear to be doing anything:
<copy todir="${dest.dir}"> <fileset dir="${src.dir}" casesensitive="yes"> <include name="**${file.separator}foo.pdf" /> </fileset> <mapper type="glob" from="foo.pdf" to="x${file.separator}foo.pdf" /> </copy>
What am I missing?
-
Admin over 13 yearsSorry, I was obfuscating my filenames to the point of confusion. They both should have been renamed foo.pdf. In any event, the first code block did it for me. Thank you! Any ideas on why my glob mapper was wrong?
-
martin clayton over 13 years@GJTorikian - I added a note on the glob mapper, hth.