Using Mockito with Retrofit 2.0
on official repository of Retrofit there's an example which can be useful: https://github.com/square/retrofit/tree/master/retrofit-mock
I've also found: https://touk.pl/blog/2014/02/26/mock-retrofit-using-dagger-and-mockito/
Here you would find this fragment:
Unit Tests
During develop of app, you can send requests the server all time(or most of time) so it is possible to live without mocked server, it sucks but is possible. Unfortunately you are not able to write good tests without the mock. Below there are two unit tests. Actually they do not test anything but in simple way shows how to mock
Retrofit
service usingMockito
andDagger
.
@RunWith(RobolectricTestRunner.class)
public class EchoServiceTest {
@Inject
protected EchoService loginService;
@Inject
protected Client client;
@Before
public void setUp() throws Exception {
Injector.add(new AndroidModule(),
new RestServicesModule(),
new RestServicesMockModule(),
new TestModule());
Injector.inject(this);
}
@Test
public void shouldReturnOfferInAsyncMode() throws IOException {
//given
int expectedQuantity = 765;
String responseContent = "{" +
" \"message\": \"mock message\"," +
" \"quantity\": \"" + expectedQuantity + "\"" +
"}";
mockResponseWithCodeAndContent(200, responseContent);
//when
EchoResponse echoResponse = loginService.getMessageAndQuantity("test", "test");
//then
assertThat(echoResponse.getQuantity()).isEqualTo(expectedQuantity);
}
@Test
public void shouldReturnOfferInAsyncModea() throws IOException {
//given
int expectedQuantity = 2;
String responseContent = "{" +
" \"message\": \"mock message\"," +
" \"quantity\": \"" + expectedQuantity + "\"" +
"}";
mockResponseWithCodeAndContent(200, responseContent);
//when
EchoResponse echoResponse = loginService.getMessageAndQuantity("test", "test");
//then
assertThat(echoResponse.getQuantity()).isEqualTo(expectedQuantity);
}
protected void mockResponseWithCodeAndContent(int httpCode, String content) throws IOException {
Response response = createResponseWithCodeAndJson(httpCode, content);
when(client.execute(Matchers.anyObject())).thenReturn(response);
}
private Response createResponseWithCodeAndJson(int responseCode, String json) {
return new Response(responseCode, "nothing", Collections.EMPTY_LIST, new TypedByteArray("application/json", json.getBytes()));
}
Read also: Square retrofit server mock for testing
Hope it help
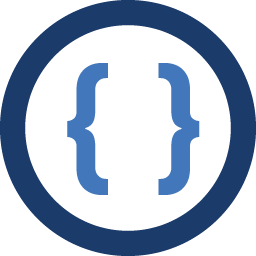
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I'm trying to create unit tests for my api calls(made via
Retrofit
2.0) usingMockito
.This seemed to be the most popular blog on using
Mockito
withRetrofit
.http://mdswanson.com/blog/2013/12/16/reliable-android-http-testing-with-retrofit-and-mockito.html
Unfortunately, it uses earlier versions of
Retrofit
, and depends on theCallbacks
andRetrofitError
, which are discontinued from 2.0.How do you do this with
Retrofit 2.0
?P.S.: I'm using
RxJava
along withretrofit
, so something that works withRxJava
would be great. Thanks!