Using mutexes/semaphores with processes
Solution 1
Just to be clear, POSIX (and linux) support two separate families of semaphores that have two different interfaces and methods of usage.
There is the older SysV semaphores consisting of semget
, semop
, semctl
and (somewhat optionally) ftok
.
The more modern "posix" semaphores consist of sem_open/sem_init
, sem_wait
, sem_post
, sem_close
and sem_unlink
.
The setup/usage regimes and capabilities are different enough that it is worth familiarizing yourself with both to see what is better for your use case.
You can also use process shared mutexes from the pthreads package.
Solution 2
Sounds like you're looking for System V IPC You would probably use a semaphore to synchronize between processes.
Here is a nice introduction to Sys V IPC in Linux
Solution 3
You'll want named mutex/semaphore's. Take a look at this StackOverflow answer that describes some points. Here's an IBM describing the use of pthread_mutexattr_setname_np
. One thing to note that named mutex's in Linux are not 100% portable (i.e. it MIGHT work on Ubuntu but NOT on CentOS, etc. etc.), so you'll need to be sure your platform supports it. If a named mutex is not available on your system, you could use named pipes with some wait conditions or even local sockets. As another answer points out is SysV IPC.
The biggest question you'll need to answer first is how 'cross-linux-platform' compatible do you want your app to be.
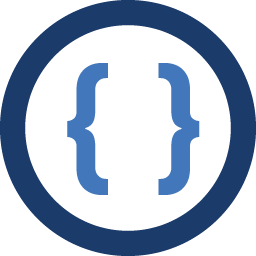
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
Almost all the code and tutorials that I have read online so far involve using mutexes and semaphores for synchronisation amongst threads. Can they be used to synchronise amongst processes?
I'd like to write code that looks like this:
void compute_and_print() { // acquire mutex // critical section // release mutex } void main() { int pid = fork(); if ( pid == 0 ) { // do something compute_and_print(); } else { // do something compute_and_print(); } }
- Could someone point me towards similar code that does this?
- I understand that different processes have different address spaces, but I wonder if the above would be different address spaces, however, wouldn't the mutex refer to the same kernel object?