Using Python to replace MATLAB: how to import data?
Solution 1
Depending on what kind of computations you are doing with MATLAB (and on which toolboxes you are using), Python could be a good alternative to MATLAB.
Python + NumPy + SciPy + Matplotlib are the right combination to start.
For the data, you can, for example, save your data directly in text file (assuming that you are not directly concerned by floating-point precision issues) and read it in Python.
If your data are Excel data, where each value is separated by a ";", you can for example read the file line by line, and use the split() method (with ";" as argument) to get each value.
For MATLAB up to version 7.1, it is possible to directly load .mat files from Python with the scipy.io.matlab.mio module.
Solution 2
Pandas is a Python data analysis library that can import/export from Excel pretty easily. Here's how to do it:
http://pandas.pydata.org/pandas-docs/stable/10min.html#excel
Crash course:
import pandas as pd
data = pd.read_excel('foo.xlsx', 'Sheet1', index_col=None, na_values=['NA'])
Solution 3
If you come from the MATLAB world, Pylab will ease your transition. Once you have converted your data to ASCII, pylab.load()
will do the rest:
pylab.load(fname, comments='#', delimiter=None, converters=None,
skiprows=0, usecols=None, unpack=False,
dtype=<type 'numpy.float64'>)
Solution 4
There's Matplotlib for plots and the csv module for reading Excel data (assuming you can dump to CSV).
Here's a tutorial about replacing MATLAB with Python.
Solution 5
If you saved you data in MATLAB format, use:
from scipy.io import loadmat
datafile = "yourfile.mat"
data = loadmat(datafile, matlab_compatible=True)
var1 = data['nameOfYourVariable'].squeeze()
var2 = data['nameOfYourOtherVariable'].squeeze()
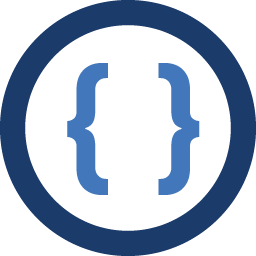
Admin
Updated on September 08, 2020Comments
-
Admin almost 4 years
I want to use some Python libraries to replace MATLAB. How could I import Excel data in Python (for example using NumPy) to use them?
I don't know if Python is a credible alternative to MATLAB, but I want to try it. Is there a a tutorial?
-
stephan almost 15 yearsSAGE (mentionend in the tutorial, see sagemath.org) is a pretty cool stack based on Python.
-
Admin almost 15 yearsThanx a lot. I'm going to try SAGE.