Using R, how to calculate the distance from one point to a line?
It has to be distinguished whether we are dealing with a two-dimensional or a three-dimensional case.
2D case
If the problem is two-dimensional, the position of the points a
, b
and c
can be defined by pairs of numbers which represent the points' x
and the y
coordinates.
The following function can be used to calculate the distance d
of the point a
from the line defined by the two points b
and c
:
dist2d <- function(a,b,c) {
v1 <- b - c
v2 <- a - b
m <- cbind(v1,v2)
d <- abs(det(m))/sqrt(sum(v1*v1))
}
Here is an example showing how the function can be applied:
## two-dimensional case:
a2 <- c(0,2)
b2 <- c(2,0)
c2 <- c(1,3)
d2 <- dist2d(a2,b2,c2) # distance of point a from line (b,c) in 2D
#> d2
#[1] 1.264911
3D case
In three dimensions, the problem is slightly more complicated. We can use the following two functions:
dist3d <- function(a,b,c) {
v1 <- b - c
v2 <- a - b
v3 <- cross3d_prod(v1,v2)
area <- sqrt(sum(v3*v3))/2
d <- 2*area/sqrt(sum(v1*v1))
}
cross3d_prod <- function(v1,v2){
v3 <- vector()
v3[1] <- v1[2]*v2[3]-v1[3]*v2[2]
v3[2] <- v1[3]*v2[1]-v1[1]*v2[3]
v3[3] <- v1[1]*v2[2]-v1[2]*v2[1]
return(v3)
}
The main function to calculate the distance can be called in the same way as in the previous example in two dimensions, with the sole difference that now the points are defined by three coordinates representing x
, y
and z
, as shown in the example below:
## three-dimensional case:
a3 <- c(0,0,2)
b3 <- c(1,0,0)
c3 <- c(2,3,1)
d3 <- dist3d(a3,b3,c3) # distance of point a from line (b,c) in 3D
#> d3
#[1] 2.215647
The equations used in this answer are described in various textbooks and can be found, e.g., here and here.
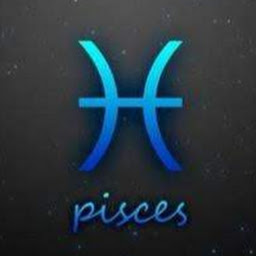
Feng Chen
Updated on July 05, 2022Comments
-
Feng Chen almost 2 years
Suppose we have three points, a, b, c. b and c are linked to become a line. how to use R to calculate the distance from a to this line? Is there any function?