Using Retrofit in Android
Solution 1
Using Retrofit is quite simple and straightforward.
First of all you need to add retrofit to your project, as example with Gradle build sytem.
compile 'com.squareup.retrofit:retrofit:1.7.1' |
another way you can download .jar and place it to your libs folder.
Then you need to define interfaces that will be used by Retrofit to make API calls to your REST endpoints. For example for users:
public interface YourUsersApi {
//You can use rx.java for sophisticated composition of requests
@GET("/users/{user}")
public Observable<SomeUserModel> fetchUser(@Path("user") String user);
//or you can just get your model if you use json api
@GET("/users/{user}")
public SomeUserModel fetchUser(@Path("user") String user);
//or if there are some special cases you can process your response manually
@GET("/users/{user}")
public Response fetchUser(@Path("user") String user);
}
Ok. Now you have defined your API interface an you can try to use it.
To start you need to create an instance of RestAdapter and set base url of your API back-end. It's also quite simple:
RestAdapter restAdapter = new RestAdapter.Builder()
.setEndpoint("https://yourserveraddress.com")
.build();
YourUsersApi yourUsersApi = restAdapter.create(YourUsersApi.class);
Here Retrofit will read your information from interface and under the hood it will create RestHandler according to meta-info your provided which actually will perform HTTP requests.
Then under the hood, once response is received, in case of json api your data will be transformed to your model using Gson library so you should be aware of that fact that limitations that are present in Gson are actually there in Retrofit.
To extend/override process of serialisers/deserialisation your response data to your models you might want to provide your custom serialisers/deserialisers to retrofit.
Here you need to implement Converter interface and implement 2 methods fromBody() and toBody().
Here is example:
public class SomeCustomRetrofitConverter implements Converter {
private GsonBuilder gb;
public SomeCustomRetrofitConverter() {
gb = new GsonBuilder();
//register your cursom custom type serialisers/deserialisers if needed
gb.registerTypeAdapter(SomeCutsomType.class, new SomeCutsomTypeDeserializer());
}
public static final String ENCODING = "UTF-8";
@Override
public Object fromBody(TypedInput body, Type type) throws ConversionException {
String charset = "UTF-8";
if (body.mimeType() != null) {
charset = MimeUtil.parseCharset(body.mimeType());
}
InputStreamReader isr = null;
try {
isr = new InputStreamReader(body.in(), charset);
Gson gson = gb.create();
return gson.fromJson(isr, type);
} catch (IOException e) {
throw new ConversionException(e);
} catch (JsonParseException e) {
throw new ConversionException(e);
} finally {
if (isr != null) {
try {
isr.close();
} catch (IOException ignored) {
}
}
}
}
@Override
public TypedOutput toBody(Object object) {
try {
Gson gson = gb.create();
return new JsonTypedOutput(gson.toJson(object).getBytes(ENCODING), ENCODING);
} catch (UnsupportedEncodingException e) {
throw new AssertionError(e);
}
}
private static class JsonTypedOutput implements TypedOutput {
private final byte[] jsonBytes;
private final String mimeType;
JsonTypedOutput(byte[] jsonBytes, String encode) {
this.jsonBytes = jsonBytes;
this.mimeType = "application/json; charset=" + encode;
}
@Override
public String fileName() {
return null;
}
@Override
public String mimeType() {
return mimeType;
}
@Override
public long length() {
return jsonBytes.length;
}
@Override
public void writeTo(OutputStream out) throws IOException {
out.write(jsonBytes);
}
}
}
And now you need to enable your custom adapters, if it was needed by using setConverter() on building RestAdapter
Ok. Now you are aware how you can get your data from server to your Android application. But you need somehow mange your data and invoke REST call in right place. There I would suggest to use android Service or AsyncTask or loader or rx.java that would query your data on background thread in order to not block your UI.
So now you can find the most appropriate place to call
SomeUserModel yourUser = yourUsersApi.fetchUser("someUsers")
to fetch your remote data.
Solution 2
I have just used retrofit for a couple of weeks and at first I found it hard to use in my application. I would like to share to you the easiest way to use retrofit in you application. And then later on if you already have a good grasp in retrofit you can enhance your codes(separating your ui from api and use callbacks) and maybe get some techniques from the post above.
In your app you have Login,Activity for list of task,and activity to view detailed task.
First thing is you need to add retrofit in your app and theres 2 ways, follow @artemis post above.
Retrofit uses interface as your API. So, create an interface class.
public interface MyApi{
/*LOGIN*/
@GET("/api_reciever/login") //your login function in your api
public void login(@Query("username") String username,@Query("password") String password,Callback<String> calback); //this is for your login, and you can used String as response or you can use a POJO, retrofit is very rubust to convert JSON to POJO
/*GET LIST*/
@GET("/api_reciever/getlist") //a function in your api to get all the list
public void getTaskList(@Query("user_uuid") String user_uuid,Callback<ArrayList<Task>> callback); //this is an example of response POJO - make sure your variable name is the same with your json tagging
/*GET LIST*/
@GET("/api_reciever/getlistdetails") //a function in your api to get all the list
public void getTaskDetail(@Query("task_uuid") String task_uuid,Callback<Task> callback); //this is an example of response POJO - make sure your variable name is the same with your json tagging
}
Create another interface class to hold all your BASE ADDRESS of your api
public interface Constants{
public String URL = "www.yoururl.com"
}
In your Login activity create a method to handle the retrofit
private void myLogin(String username,String password){
RestAdapter restAdapter = new RestAdapter.Builder()
.setEndpoint(Constants.URL) //call your base url
.build();
MyApi mylogin = restAdapter.create(MyApi.class); //this is how retrofit create your api
mylogin.login(username,password,new Callback<String>() {
@Override
public void success(String s, Response response) {
//process your response if login successfull you can call Intent and launch your main activity
}
@Override
public void failure(RetrofitError retrofitError) {
retrofitError.printStackTrace(); //to see if you have errors
}
});
}
In your MainActivityList
private void myList(String user_uuid){
RestAdapter restAdapter = new RestAdapter.Builder()
.setEndpoint(Constants.URL) //call your base url
.build();
MyApi mytask = restAdapter.create(MyApi.class); //this is how retrofit create your api
mytask.getTaskDetail(user_uuid,new Callback<Task>>() {
@Override
public void success(ArrayList<Task> list, Response response) {
//process your response if successful load the list in your listview adapter
}
@Override
public void failure(RetrofitError retrofitError) {
retrofitError.printStackTrace(); //to see if you have errors
}
});
}
In your Detailed List
private void myDetailed(String task_uuid){
RestAdapter restAdapter = new RestAdapter.Builder()
.setEndpoint(Constants.URL) //call your base url
.build();
MyApi mytask = restAdapter.create(MyApi.class); //this is how retrofit create your api
mytask.getTaskList(task_uuid,new Callback<Task>() {
@Override
public void success(Task task, Response response) {
//process your response if successful do what you want in your task
}
@Override
public void failure(RetrofitError retrofitError) {
retrofitError.printStackTrace(); //to see if you have errors
}
});
}
Hope this would help you though its really the simplest way to use retrofit.
Solution 3
Take a look at this , excellent blog on using Retrofit in conjunction with Otto, both libraries are from Square.
http://www.mdswanson.com/blog/2014/04/07/durable-android-rest-clients.html
The basic idea is that you will hold a reference to a "repository" object in your Application class. This object will have methods that "subscribe" to rest api event requests. When one is received it will make the proper Retrofit call, and then "post" the response, which can then be "subscribed" to by another component (such as the activity that made the request).
Once you have this all setup properly, accessing data via your rest api becomes very easy. For example, making are request for data would look something like this :
mBus.post(new GetMicropostsRequest(mUserId));
and consuming the data would look something like this:
@Subscribe
public void onGetUserProfileResponse(GetUserProfileResponse event) {
mView.setUserIcon("http://www.gravatar.com/avatar/" + event.getGravatar_id());
mView.setUserName(event.getName());
}
It takes a little bit of upfront effort, but in the end it becomes "trivial" to access anything you need from our backend via Rest.
Solution 4
You may try saving references to your api inside your application class. Then you can get it's instance from any activity or fragment and get api from there. That sounds a little weird, but it may be a simple DI alternative. And if you will only store references in your app class, it won't be a kind of god object
UPD: http://square.github.io/retrofit/ - here is some documentation, it might be useful
Solution 5
Using RetroFit is very easy.
-
Add dependecy in build.gradle.
compile 'com.squareup.retrofit:retrofit:1.9.0' compile 'com.squareup.okhttp:okhttp:2.4.0'
Make an Interface for all http methods.
Copy your json output and create pojo class to recieve json of your
response, you can make pojo from JsonSchema2pojo site .-
make an adapter and call your method
for complete demo try this tutorial Retrofit Android example
Related videos on Youtube
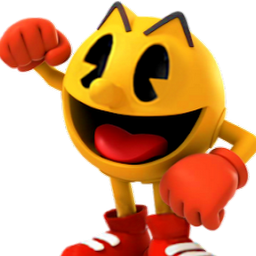
Comments
-
Stack Man almost 2 years
I have an android app that has 3 activities :
- A login activity
- A tasks acivity where all tasks pertaining to a user are displayed (Populated using an Array Adapter)
- A task_details activity which results from clicking a task on the list
I have to consume REST Apis. The research I have done so far directs me to use Retrofit. I checked how to use it and found out that :
- Set the base URL in the Main Activity (Mine is the Login Activity)
- I need to create a API class and define my functions using annotations.
- Use the class Rest Adapter in the Activity and define Callbacks.
Had my app been a single activity app, I would have crunched everything in my MainActivity.java but I don't know how and where to put all the code from steps 1,2,3 for use in my 3 activities.Could you please help by telling how to use Retrofit in my app. Thanks a lot.
Specifically, I need network calls to : 1. Login the user 2. Get all the tasks of the user. And for both I would be using a given REST api.
********************************************* Calling Api USing Retrofit ********************************************* **Dependancies** :- implementation 'com.android.support:recyclerview-v7:27.1.1' implementation 'com.squareup.picasso:picasso:2.5.2' implementation 'com.android.support:cardview-v7:27.1.1' enter code here **Model** use the Pozo class **Api Call** -> getLogin() // use the method //API call for Login private void getLogin() { getWindow().setFlags(WindowManager.LayoutParams.FLAG_NOT_TOUCHABLE, WindowManager.LayoutParams.FLAG_NOT_TOUCHABLE); AsyncHttpClient client = new AsyncHttpClient(); RequestParams requestParams = new RequestParams(); requestParams.put("email_id", edit_email.getText().toString()); requestParams.put("password", edit_password.getText().toString()); Log.e("", "LOGIN URL==>" + Urls.LOGIN + requestParams); Log.d("device_token", "Device_ Token" + FirebaseInstanceId.getInstance().getToken()); client.post(Urls.LOGIN, requestParams, new JsonHttpResponseHandler() { @Override public void onStart() { super.onStart(); ShowProgress(); } @Override public void onFinish() { super.onFinish(); Hideprogress(); } @Override public void onSuccess(int statusCode, Header[] headers, JSONObject response) { super.onSuccess(statusCode, headers, response); Log.e("", "Login RESPONSE-" + response); Login login = new Gson().fromJson(String.valueOf(response), Login.class); edit_email.setText(""); edit_password.setText(""); if (login.getStatus().equals("true")) { getWindow().clearFlags(WindowManager.LayoutParams.FLAG_NOT_TOUCHABLE); MDToast mdToast = MDToast.makeText(SignInActivity.this, String.valueOf("User Login Successfully!"), MDToast.LENGTH_SHORT, MDToast.TYPE_SUCCESS); mdToast.show(); Utils.WriteSharePrefrence(SignInActivity.this, Util_Main.Constant.EMAIL, login.getData().getEmailId()); Utils.WriteSharePrefrence(SignInActivity.this, Constant.USERID, login.getData().getId()); Utils.WriteSharePrefrence(SignInActivity.this, Constant.USERNAME, login.getData().getFirstName()); Utils.WriteSharePrefrence(SignInActivity.this, Constant.PROFILE, login.getData().getProfileImage()); hideKeyboard(SignInActivity.this); Intent intent = new Intent(SignInActivity.this, DashboardActivity.class); intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK); startActivity(intent); finish(); } else { getWindow().clearFlags(WindowManager.LayoutParams.FLAG_NOT_TOUCHABLE); MDToast mdToast = MDToast.makeText(SignInActivity.this, String.valueOf("Login Denied"), MDToast.LENGTH_SHORT, MDToast.TYPE_ERROR); mdToast.show(); } } @Override public void onFailure(int statusCode, Header[] headers, String responseString, Throwable throwable) { super.onFailure(statusCode, headers, responseString, throwable); Log.e("", throwable.getMessage()); getWindow().clearFlags(WindowManager.LayoutParams.FLAG_NOT_TOUCHABLE); MDToast mdToast = MDToast.makeText(SignInActivity.this, "Something went wrong", MDToast.LENGTH_SHORT, MDToast.TYPE_ERROR); mdToast.show(); } }); }
-
Stack Man over 9 yearsI don't find enough documentations/tutorials for using Retrofit.
-
Rafed Nole over 9 yearsDid you get it resolved ?
-
Pankaj Arora over 9 yearsbetter to use volley for fast networking.
-
peitek about 9 yearsA little late to the party, but for future searchers: you can find a fairly extensive guide for retrofit here.
-
Rock Lee over 8 years
Unresolved reference: RestAdapter
It appears RestAdapter is no longer used in version 2. This question helped explain that: stackoverflow.com/questions/32424184/… -
Mohsen Kamrani over 8 yearsExcuse me if my question is very trivial but I'm really struggling to start using Retrofit (yet zero progress). Should we have something like a php page to accomplish the task?
-
chkm8 over 8 yearsRetrofit is a REST api for android, basically you need to have a server side application, like php where you will need also to build your api. If you havent tried to create a simple mobile application with REST in android, you might start with this tutorial,androidhive.info/2012/01/…. And if you already have experience in REST, Retrofit will be a good library to use.
-
Mohsen Kamrani over 8 yearsThank you. Indeed I've already tried this and I can understand it. You create a php page which receives what you have send it as POST and generates the response in JSON format. Do you anything even close to that tutorial for retrofit?
-
chkm8 over 8 yearsIt is not a php page actually., it should be an api, normally plain php script that accepts request like POST/GET in JSON/XML format. Here is an example of api. function login_post(){ $email = $this->post('email'); $password = $this->post('password'); $data = $this->Auth_model->authenticate($email, $password); print_r($data); }
-
Kai Wang over 7 yearsthis is retrofit 1. people are using retrofit 2 now. the syntax is different.
-
Vinayak Mestri over 3 yearsEasy way to add retrofit in your project with just one click. (one time setup) medium.com/@mestri.vinayak.n/…