Using round() function in c
Solution 1
The wording in the man-page is meant to be read literally, that is in its mathematical sense. The wording "x is integral" means that x is an element of Z, not that x has the data type int
.
Casting a double
to int
can be dangerous because the maximum arbitrary integral value a double
can hold is 2^52 (assuming an IEEE 754 conforming binary64 ), the maximum value an int
can hold might be smaller (it is mostly 32 bit on 32-bit architectures and also 32-bit on some 64-bit architectures).
If you need only powers of ten you can test it with this little program yourself:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main(){
int i;
for(i = 0;i < 26;i++){
printf("%d:\t%.2f\t%d\n",i, pow(10,i), (int)pow(10,i));
}
exit(EXIT_SUCCESS);
}
Instead of casting you should use the functions that return a proper integral data type like e.g.: lround(3)
.
Solution 2
here is an excerpt from the man page.
#include <math.h>
double round(double x);
float roundf(float x);
long double roundl(long double x);
notice: the returned value is NEVER a integer. However, the fractional part of the returned value is set to 0.
notice: depending on exactly which function is called will determine the type of the returned value.
Here is an excerpt from the man page about which way the rounding will be done:
These functions round x to the nearest integer, but round halfway cases
away from zero (regardless of the current rounding direction, see
fenv(3)), instead of to the nearest even integer like rint(3).
For example, round(0.5) is 1.0, and round(-0.5) is -1.0.
Solution 3
If you want a long integer to be returned then please use lround
:
long int tolongint(double in)
{
return lround(in));
}
For details please see lround which is available as of the C++ 11 standard.
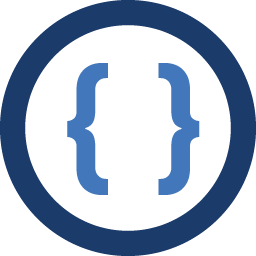
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm a bit confused about the
round()
function in C.First of all, man says:
SYNOPSIS #include <math.h> double round(double x); RETURN VALUE These functions return the rounded integer value. If x is integral, +0, -0, NaN, or infinite, x itself is returned.
The return value is a
double
/float
or anint
?In second place, I've created a function that first rounds, then casts to int. Latter on my code I use it as a mean to compare doubles
int tointn(double in,int n) { int i = 0; i = (int)round(in*pow(10,n)); return i; }
This function apparently isn't stable throughout my tests. Is there redundancy here? Well... I'm not looking only for an answer, but a better understanding on the subject.
-
Admin over 7 yearsThanks, I wasn't aware about lround(). I will try it.
-
Admin over 7 years"However, the fractional part of the returned value is set to 0." In which precision that's true?
-
Admin over 7 yearsNice that you've mentioned the "halfway cases". What about the fact that 0.5 could be either 0.5000001 or 0.49999998 for example? How does 'round()' deal with that?
-
user3629249 over 7 years@Mattana, strongly suggest reading the man page for
round()
to get all the details/ ALL the part to the right of the decimal is set to 0 regardless of the 'precision' used in displaying the value. That is one of the things thatround()
does. a value with less than .5 will be rounded toward 0, like thefloor()
function. a value with greater than .5 will be rounded toward infinity, like theceil()
function. Besure to note the slight difference on the cases when the overall number is negative