Using scanf to read in certain amount of characters in C?
Solution 1
Normally, you'd use something like "%4c"
or "%4s"
to read a maximum of 4 characters (the difference is that "%4c"
reads the next 4 characters, regardless, while "%4s"
skips leading whitespace and stops at a whitespace if there is one).
To specify the length at run-time, however, you have to get a bit trickier since you can't use a string literal with "4" embedded in it. One alternative is to use sprintf
to create the string you'll pass to scanf
:
char buffer[128];
sprintf(buffer, "%%%dc", max_length);
scanf(buffer, your_string);
I should probably add: with printf
you can specify the width or precision of a field dynamically by putting an asterisk (*
) in the format string, and passing a variable in the appropriate position to specify the width/precision:
int width = 10;
int precision = 7;
double value = 12.345678910;
printf("%*.*f", width, precision, value);
Given that printf
and scanf
format strings are quite similar, one might think the same would work with scanf
. Unfortunately, this is not the case--with scanf
an asterisk in the conversion specification indicates a value that should be scanned, but not converted. That is to say, something that must be present in the input, but its value won't be placed in any variable.
Solution 2
Try
scanf("%4s", str)
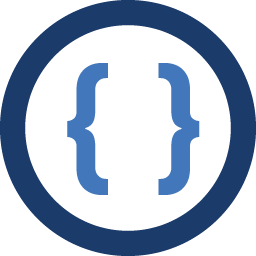
Admin
Updated on June 17, 2022Comments
-
Admin almost 2 years
I am having trouble accepting input from a text file. My program is supposed to read in a string specified by the user and the length of that string is determined at runtime. It works fine when the user is running the program (manually inputting the values) but when I run my teacher's text file, it runs into an infinite loop.
For this example, it fails when I am taking in 4 characters and his input in his file is "ABCDy". "ABCD" is what I am supposed to be reading in and 'y' is supposed to be used later to know that I should restart the game. Instead when I used scanf to read in "ABCD", it also reads in the 'y'. Is there a way to get around this using scanf, assuming I won't know how long the string should be until runtime?