Using Sikuli to verify text
Rather than using exists() to verify text (because the OCR in sikuli's IDE is pretty unreliable), if there is any way to get the text that you want to evaluate to the clipboard, you can use Env.getClipboard() to evaluate it with much more accuracy.
To get it to the clipboard, you could use several approaches:
- dragDrop() to highlight the text
- Perhaps tabbing into the text box will highlight the text for you automatically
- Use doubleClick() on the text (depending on what you're trying to highlight, thus might not get it all)
- Perhaps the most reliable way to highlight it--tab into the text box or click() inside the text box and select all:
.
click(someImageNearTextBox).offset() #get your cursor inside the textbox
type("a",KeyModifier.CTRL) #select all to highlight the text
Once your text is highlighted, you can proceed like this:
type("c",KeyModifier.CTRL) #copy selection to the clipboard
firstName = Env.getClipboard().strip() #assign contents of clipboard to a variable
Then you can use it for whatever comparisons you want:
if firstName == "FirstName01":
popup('passed')
else:
popup('failed')
The weakness of this approach is that if you have any special characters in the textbox, it may not evaluate properly.
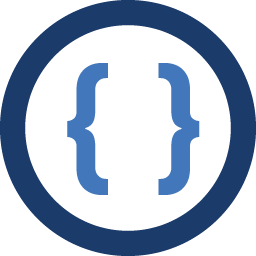
Admin
Updated on June 23, 2022Comments
-
Admin almost 2 years
I am using the Sikuli IDE to test an application that returns data in a text box. For example I search the name field for my test value 'FirstName01' the application returns the name and address in various text boxes.
I then verify the data by using the exists() function in Sikuli. To do this I click on the exists function in the upper left of the IDE and use the + tool to select the text I want to verify. In this case FirstName01 and Location01. I then set the Similarity setting on the MatchingPreview tab to .98 (I find if I set it to 1.0 Sikuli fails the test even if I get the correct data back).
If I run the test searching for FirstName01 I get correct results back and Sikuli does not throw an error. My problem is if I search for and return FirstName02 in an attempt to generate the error condition the exists function passes it even though it is looking for FirstName01. It seems Sikuli does not verify the last character of the data. It seems to verify other characters because if I search for FirstName21 the exists function throws an error as it should. Has anyone come across this problem and if so how did you solve it?
My code is below
If exists(FirstName01): popup('passed') else: popup('failed')
Is there another way to verify data?