Using single Timer vs multiple Timers for scheduling TimerTasks in Android
The first solution creates one timer object; the second one multiple timers. As the javadoc clearly explains, each timer comes with its own thread:
Corresponding to each Timer object is a single background thread that is used to execute all of the timer's tasks, sequentially. Timer tasks should complete quickly. If a timer task takes excessive time to complete, it "hogs" the timer's task execution thread. This can, in turn, delay the execution of subsequent tasks, which may "bunch up" and execute in rapid succession when (and if) the offending task finally completes.
Thus: the second solution guarantees that there are three threads for your three tasks. In other words: you use more resources, but as a result of doing so, you can be sure that your different tasks are not "blocking" each other (when two tasks need to be run on the same thread, well: it is only one thread; it can't execute two tasks in parallel!)
But beyond that: when I read around the many answers that a simple search for "java timer android" shows, the real answers seems to be: on Android, you should prefer to use a Handler instead of Timer objects.
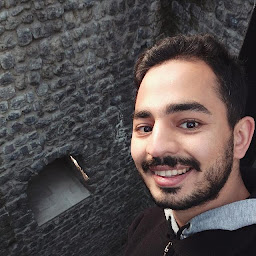
Safa Kadir
Software Development Engineer at Telenity EMEA Java, Node.js, Vue, Mongo. Fullstack web development where backend takes more presedence. Bachelor of Computer Engineering and Electirical-Electronics Engineering degrees. Also had a little Master of Science adventure.
Updated on June 20, 2022Comments
-
Safa Kadir almost 2 years
I think I don't fully understand how the Timer and TimerTask work in Java and Android. Now, I have a number of periodic tasks defined, to be scheduled within a timer.
I wonder should I use a single timer to schedule tasks, or using different timer instances for each task is ok? Do timers have their own threads? Do scheduled tasks executed in new threads? What is happening in the background?
What is the differences between these approaches?
Sample code for approach 1:
private void initTasks() { TimerTask task1 = new MyTimerTask1(); TimerTask task2 = new MyTimerTask2(); TimerTask task3 = new MyTimerTask3(); Timer timer = new Timer(); timer.schedule(task1, 0, TASK1_PERIOD); timer.schedule(task2, 0, TASK2_PERIOD); timer.schedule(task3, 0, TASK3_PERIOD); } class MyTimerTask1 extends TimerTask { public void run() { //do task1 } } class MyTimerTask2 extends TimerTask { public void run() { //do task2 } } class MyTimerTask3 extends TimerTask { public void run() { //do task3 } }
Sample code for approach 2:
private void initTasks() { MyTimerTask1 task1 = new MyTimerTask1(); MyTimerTask2 task2 = new MyTimerTask2(); MyTimerTask3 task3 = new MyTimerTask3(); task1.start(); task2.start(); task3.start(); } class MyTimerTask1 extends TimerTask { private Timer timer; public void run() { //do task1 } public void start() { timer = new Timer(); timer.schedule(this, 0, TASK1_PERIOD); } } class MyTimerTask2 extends TimerTask { private Timer timer; public void run() { //do task2 } public void start() { timer = new Timer(); timer.schedule(this, 0, TASK2_PERIOD); } } class MyTimerTask3 extends TimerTask { private Timer timer; public void run() { //do task3 } public void start() { timer = new Timer(); timer.schedule(this, 0, TASK3_PERIOD); } }