using Tor as Proxy
Solution 1
If you have privoxy installed and running you can do
request.Proxy = new WebProxy("127.0.0.1:8118"); // default privoxy port
Which will enable you to make requests using tor
Solution 2
Tor is not an HTTP proxy. It's a SOCKS proxy. You can use an HTTP proxy that supports forwarding on SOCKS (like Privoxy) and connect to that via code instead.
Solution 3
Yes like the other poster said, a socks client is needed. Some libraries are Starksoft Proxy, ProxySocket and ComponentSpace Socks Proxy. sockscap is a tool that intercepts and reroutes winsock calls, and privoxy is a local proxy that can tunnel your requests over socks. A couple different solutions.
Solution 4
Use the library "SocksWebProxy". You can use it with WebClient & WebRequest (Just assign a new SocksWebProxy to the *.Proxy attribute). No Need for Privoxy or similar service to translate http traffic to tor.
https://github.com/Ogglas/SocksWebProxy
I made some extensions to it as well by enabling the control port. Here is how you could have Tor running in the background without Tor Browser Bundle started and to control Tor we can use Telnet or send commands programmatically via Socket.
Socket server = null;
//Authenticate using control password
IPEndPoint endPoint = new IPEndPoint(IPAddress.Parse("127.0.0.1"), 9151);
server = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
server.Connect(endPoint);
server.Send(Encoding.ASCII.GetBytes("AUTHENTICATE \"your_password\"" + Environment.NewLine));
byte[] data = new byte[1024];
int receivedDataLength = server.Receive(data);
string stringData = Encoding.ASCII.GetString(data, 0, receivedDataLength);
//Request a new Identity
server.Send(Encoding.ASCII.GetBytes("SIGNAL NEWNYM" + Environment.NewLine));
data = new byte[1024];
receivedDataLength = server.Receive(data);
stringData = Encoding.ASCII.GetString(data, 0, receivedDataLength);
if (!stringData.Contains("250"))
{
Console.WriteLine("Unable to signal new user to server.");
server.Shutdown(SocketShutdown.Both);
server.Close();
}
else
{
Console.WriteLine("SIGNAL NEWNYM sent successfully");
}
Steps to configure Tor:
- Copy torrc-defaults into the directory in which tor.exe is. Default directory if you are using Tor browser is: "~\Tor Browser\Browser\TorBrowser\Data\Tor"
- Open a cmd prompt window
- chdir to the directory where tor.exe is. Default directory if you are using Tor browser is: "~\Tor Browser\Browser\TorBrowser\Tor\"
- Generate a password for Tor control port access.
tor.exe --hash-password “your_password_without_hyphens” | more
- Add your password password hash to torrc-defaults under ControlPort 9151. It should look something like this:
hashedControlPassword 16:3B7DA467B1C0D550602211995AE8D9352BF942AB04110B2552324B2507
. If you accept your password to be "password" you can copy the string above. - You can now access Tor control via Telnet once it is started. Now the code can run, just edit the path to where your Tor files are located in the program. Test modifying Tor via Telnet:
- Start tor with the following command:
tor.exe -f .\torrc-defaults
- Open up another cmd prompt and type:
telnet localhost 9151
- If all goes well you should see a completely black screen. Type "
autenticate “your_password_with_hyphens”
" If all goes well you should see "250 OK". - Type "
SIGNAL NEWNYM
" and you will get a new route, ergo new IP. If all goes well you should see "250 OK". - Type "
setevents circ
" (circuit events) to enable console output - Type "
getinfo circuit-status
" to see current circuits
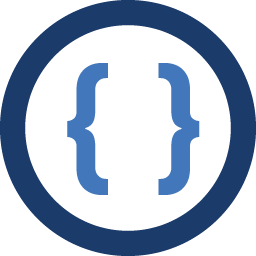
Admin
Updated on September 13, 2020Comments
-
Admin over 3 years
I'm trying to use Tor-Server as a proxy in
HttpWebRequest
, my code looks like this:HttpWebRequest request; HttpWebResponse response; request = (HttpWebRequest)WebRequest.Create("http://www.google.com"); request.Proxy = new WebProxy("127.0.0.1:9051"); response = (HttpWebResponse)request.GetResponse(); response.Close();
it works perfect with "normal" proxies but with Tor I'm getting Exceptions while calling
GetResponse() with Status = ServerProtocolViolation. The message is (in German...):Message = "Der Server hat eine Protokollverletzung ausgeführt.. Section=ResponseStatusLine"
-
mmx over 14 yearsLay: nothing is built in .NET to forward HTTP stuff via a SOCKS proxy.
-
Junior Mayhé over 12 yearsOf course you have to edit config.txt in Privoxy folder file first and uncomment the line
forward-socks5 / 127.0.0.1:9050 .
-
Marcello Grechi Lins about 10 yearsVidalia was now removed from the Tor Bundle.
-
Marcello Grechi Lins about 10 yearsI have manually downloaded and installed both the Tor Bundle and the Privoxy. I have uncomented the line mentioned on the config file and added the proxy to my webrequest object, but it gives me error 503 - server unavailable and theres no log on the privoxy indicating that the request got routed through it Have you managed to make it work?
-
confusedMind almost 10 yearsI am trying to change config.txt but it say's access denied?
-
André C. Andersen almost 10 years@confusedMind Simply open your text editor as administrator, then find the config file and edit it.
-
jamesbar2 over 9 yearsI was able to change the configuration file, after opening as an admin. However, now that I have changed these settings with Tor open, I'm receiving the same 503 error. And Provixy startup log says "000000a0 Error: Wrong number of parameters for forward-socks4a directive in configuration file." All my forward-socks4a are disabled. Thoughts?