Using WebView in Fragment
60,726
Things to do like
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View v=inflater.inflate(R.layout.fragment_bugtracker, container, false);
mWebView = (WebView) v.findViewById(R.id.webview);
mWebView.loadUrl("https://google.com");
// Enable Javascript
WebSettings webSettings = mWebView.getSettings();
webSettings.setJavaScriptEnabled(true);
// Force links and redirects to open in the WebView instead of in a browser
mWebView.setWebViewClient(new WebViewClient());
return v;
}
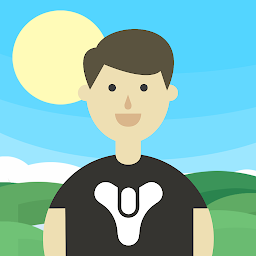
Comments
-
tomaset22 almost 2 years
recently I started creating an Android app with WebView. The app has grown and I changed the activity with WebView to tabbed activity using fragments and ViewPager. And there started my issue. After some headaches I finally managed how to display proper fragments for each tab. Then I simply copied the code for WebView to one of the fragment but the fragment isn't showing the web page I want. Any help will be appreciated!
Here's the code:
fragment with webView
import android.os.Bundle; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.webkit.WebSettings; import android.webkit.WebView; import android.webkit.WebViewClient; public class WebViewFragment extends Fragment { public WebView mWebView; @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { mWebView = (WebView) mWebView.findViewById(R.id.webview); mWebView.loadUrl("google.com"); // Enable Javascript WebSettings webSettings = mWebView.getSettings(); webSettings.setJavaScriptEnabled(true); // Force links and redirects to open in the WebView instead of in a browser mWebView.setWebViewClient(new WebViewClient()); return inflater.inflate(R.layout.fragment_bugtracker, container, false); } }
and xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".Tabs$BugtrackerFragment"> <WebView android:id="@+id/webview" android:layout_width="match_parent" android:layout_height="match_parent" /> </RelativeLayout>
-
tomaset22 almost 9 yearsNice, but I don't understand one thing, why is defining and returning
v
something else than just returning the definition of it? -
MohanRaj S over 7 years'v' denote here the View which is inflate. that is user defined variable.