Validate array of objects in express validator
20,297
Solution 1
let arr = [
{
user_id:1,
hours:8
},
{
user_id:2,
hours:7
}
]
You can put check like this, note that arr would be the key in the request body. :
check("arr.*.user_id")
.not()
.isEmpty()
check("arr.*.hours")
.not()
.isEmpty()
Solution 2
I was able to do it with wildcards like this:
app.post('/users', [
body().isArray(),
body('*.user_id', 'user_idfield must be a number').isNumeric(),
body('*.hours', 'annotations field must a number').exists().isNumeric(),
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(422).json({errors: errors.array()});
}
return res.status(200).json(req.body)
Solution 3
You achieve this by accessing the request body:
const { body } = require('express-validator')
body('*.*')
.notEmpty()
Solution 4
For anyone who wants to validate an array of objects using checkSchema, here's an example where I am validating an array of objects which can have 0 items. You could even do custom validation if you throw a function to do so:
JSON:
{
"employees": [
{
"roleId": 3,
"employeeId": 2,
},
{
"roleId": 5,
"employeeId": 4,
},
]
}
checkSchema:
const customValidator = (async (employees, { req }) => {
if (!Array.isArray(employees)) {
return true; // let the base isArray: true validation take over.
}
if(!something) {
throw Error('employee error');
}
// validate at your will here.
return true;
}
checkSchema({
employees: {
isArray: true,
min: 0,
custom: {
options: customValidator
},
},
"employees.*.roleId": {
isInt: true
},
"employees.*.employeeId": {
isInt: true
}
})
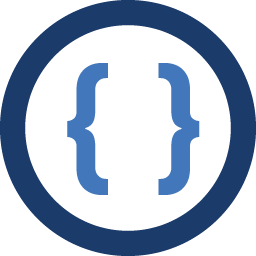
Author by
Admin
Updated on October 21, 2021Comments
-
Admin over 2 years
I am using express validator to validate my fields. But now i have array of 2 or 3 objects, which contains the "userId" and "Hours" fields like below.
[ { user_id:1, hours:8 }, { user_id:2, hours:7 } ]
Now i need to validate, if any of object property like hours or user_id is empty or not.if empty throw it's error.