validate json against schema in javascript
Solution 1
Simply, no.
There was something called JSON Schema, which was an Internet Draft which expired in 2013. Internet Drafts are the first stage to producing an Internet Standard. See more about it at the official site, as it seems to potentially still be actively developed, although it is not (to my knowledge) in widespread use.
An example of the schema:
{
"$schema": "http://json-schema.org/schema#",
"title": "Product",
"type": "object",
"required": ["id", "name", "price"],
"properties": {
"id": {
"type": "number",
"description": "Product identifier"
},
"name": {
"type": "string",
"description": "Name of the product"
},
"price": {
"type": "number",
"minimum": 0
},
"tags": {
"type": "array",
"items": {
"type": "string"
}
},
"stock": {
"type": "object",
"properties": {
"warehouse": {
"type": "number"
},
"retail": {
"type": "number"
}
}
}
}
}
will validate this example JSON:
{
"id": 1,
"name": "Foo",
"price": 123,
"tags": [
"Bar",
"Eek"
],
"stock": {
"warehouse": 300,
"retail": 20
}
}
EDIT As they all (more or less) do the same thing and have very similar syntaxes, performance should be of the biggest concern. See here for a comparison of JSON validator's performance - the winner is ajv, which is personally what I use for this reason.
Solution 2
There seems to be at least one pure JS solution now (https://github.com/tdegrunt/jsonschema) available via npm (https://www.npmjs.com/package/jsonschema). I am not a contributor, although I appreciate their work.
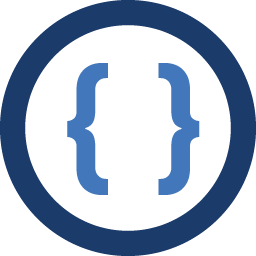
Admin
Updated on January 06, 2021Comments
-
Admin over 3 years
Question:
Is there a plain or native javascript way to validate a JSON script against a JSON schema?
I have found lots of libraries on Github, but no native/plain solution. Does EcmaScript not have a specification for this? and do none of the browsers (or nodejs) have a way to validate JSON natively?
Context of Question:
I have a very complex schema that I developed. It is supposed to work along with a script that requires that the JSON data passed into it to comply with the schema.