variable has private access
Solution 1
private int x, y, width, height;
means that they can only be accessed from the actual class where they are declared. You should create appropriate get
and set
methods and use them. You want the fields to be either public
or protected
to access them using the dot notation, but IMO it's a better design to keep them private and use get
and set
. See also In Java, difference between default, public, protected, and private that explains the fields' visibility.
Solution 2
g.setColor(color);
g.fillOval(x, y, width, height);
All these fields are private to the parent class.
You cannot access a private field from outside the class, not even from subclasses.
You could change the field to protected or provide getters for the subclasses to call.
So in Shape, add a method
protected Color getColor(){ return color; }
and then you can do
g.setColor(getColor());
in your subclasses. Same for the other fields.
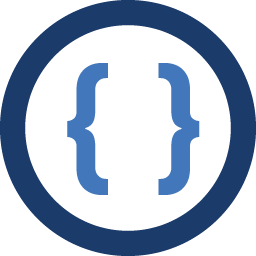
Admin
Updated on May 05, 2020Comments
-
Admin about 4 years
I'm trying to make an abstract shape class for rectangle and ellipse the only abstract method I gave shape was the draw method, but after I gave it a constructor and everything it gives me an error in the rectangle class saying that color and the other variables have private accesses here is my code:
public abstract class Shape{ private int x, y, width, height; private Color color; public Shape(int x, int y, int width, int height, Color color){ setXY(x, y); setSize(width, height); setColor(color); } public boolean setXY(int x, int y){ this.x=x; this.y=y; return true; } public boolean setSize(int width, int height){ this.width=width; this.height=height; return true; } public boolean setColor(Color color){ if(color==null) return false; this.color=color; return true; } public abstract void draw(Graphics g); } class Rectangle extends Shape{ public Rectangle(int x, int y, int width, int height, Color color){ super(x, y, width, height, color); } public void draw(Graphics g){ setColor(color); fillRect(x, y, width, height); setColor(Color.BLACK); drawRect(x, y, width, height); } } class Ellipse extends Shape{ public Ellipse(int x, int y, int width, int height, Color color){ super(x, y, width, height, color); } public void draw(Graphics g){ g.setColor(color); g.fillOval(x, y, width, height); g.setColor(Color.BLACK); g.drawOval(x, y, width, height); } }