VB.NET Renaming File and Retagging / Edit Image MetaData / Meta Tags
EDIT: This isn't a dll library, you just copy the source code to your project and create a new instance of the object.
I use a class called ExifWorks, found here: http://www.codeproject.com/KB/vb/exif_reader.aspx?msg=1813077 It's usage is simple,
Dim EX As New ExifWorks(bitmap)
Dim dateStr As String = EX.DateTimeOriginal
Dim description As String = EX.Description
EX.SetPropertyString(ExifWorks.TagNames.ImageDescription, "my description")
This is the easiest way I've found so far. Let me know if you run into any problems.
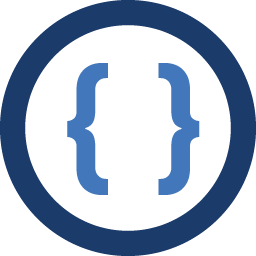
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
Clarifiration:
How do I Edit and Save Image EXIF / Metadata / FileInfo without using an external DLL?
Project:
I'm building an app for personal use to rename, retag, and organize the apocalyptic quantity of images I host on my personal website. As I have been collecting funny pictures and such for several years, there is no real rhyme or reason to the file naming conventions. Ergo, Image0001.jpg needs to be renamed to a descriptive filename, and the Metadata fields need to be filled in.
The desired process will take an existing jpg, gif, png, tiff or bmp and do the following:
- load image into memory
- convert bmp files to jpgs if needed (for a smaller file size, mostly)
- load image tags into ImageData Structure (see below)
- load file data into ImageData Structure (where needed)
- display image and tags for user to edit (In a Picture Box and several Text Boxes)
- allow editing of fields and renaming of the file
- write the changes to the image file
- go to next file.
Example:
- Load Image0001.jpg. Populate ImageData Structure fields.
- Type in Description: "lolcat ceiling cat sends son".
- ImageData.FileName changed to "lolcat-ceiling-cat-sends-son.jpg".
- ImageData.Name, .Keywords, .Title, .Subject, and .Comments changed to "lolcat ceiling cat sends son".
- Save file with new filename and save all new tag fields.
(Later, I will also be using SQL to build a referential database with links to the online copies of these files to allow for searching by keywords, subject, filename, etc, but that's another layer that's much easier than this one. At least to me.)
Problem:
So far, several days of research have yielded almost no measurable progress. Information has apparently been inexplicably hidden behind a bunch of unexpected search keywords that I have not though to use for my searches. Any help would be appreciated.
Current Code as is:
Imports System.IO Imports System.IO.Path Imports System.Drawing.Imaging Imports ImageData '(The Custom Structure below)' '*Also has a project level reference to the dso.dll referenced below.' Public Structure ImageData Shared FileAuthorAuthor As String Shared FileAuthorCategory As String Shared FileAuthorComments As String Shared FileAuthorCompany As String Shared FileAuthorDateCreated As DateTime Shared FileAuthorDescription As String Shared FileAuthorHeight As Decimal Shared FileAuthorHeightResolution As Decimal Shared FileAuthorImage As Image Shared FileAuthorKeywords As String Shared FileAuthorName As String Shared FileAuthorPath As String 'URL or IRL' Shared FileAuthorRead As Boolean Shared FileAuthorSubject As String Shared FileAuthorTitle As String Shared FileAuthorType As String Shared FileAuthorWidth As Decimal Shared FileAuthorWidthResolution As Decimal End Structure 'ImageData
And the current method for finding the data is:
Shared Function ReadExistingData(ByRef FileWithPath As String) As Boolean 'Extract the FileName' Dim PathParts As String() = FileWithPath.Split("\") '" Dim FileName As String = PathParts(PathParts.Length - 1) Dim FileParts As String() = FileName.Split(".") Dim FileType As String = FileParts(FileParts.Length - 1) 'Create an Image object. ' Dim SelectedImage As Bitmap = New Bitmap(FileWithPath) 'Get the File Info from the Image.' Dim ImageFileInfo As New FileInfo(FileWithPath) Dim dso As DSOFile.OleDocumentProperties dso = New DSOFile.OleDocumentProperties dso.Open(FileWithPath.Trim, True, DSOFile.dsoFileOpenOptions.dsoOptionOpenReadOnlyIfNoWriteAccess) ImageData.FileAuthor = dso.SummaryProperties.Author '* Requires dso.DLL' ImageData.FileCategory = dso.SummaryProperties.Category '* Requires dso.DLL' ImageData.FileComments = dso.SummaryProperties.Comments '* Requires dso.DLL' ImageData.FileCompany = dso.SummaryProperties.Company '* Requires dso.DLL' ImageData.FileDateCreated = ImageFileInfo.CreationTime ImageData.FileDescription = dso.SummaryProperties.Comments '* Requires dso.DLL.' ImageData.FileHeight = SelectedImage.Height ImageData.FileHeightResolution = SelectedImage.VerticalResolution ImageData.FileImage = New Bitmap(FileWithPath) ImageData.FileKeywords = dso.SummaryProperties.Keywords '* Requires dso.DLL' ImageData.FileName = FileName ImageData.FilePath = FileWithPath ImageData.FileRead = ImageFileInfo.IsReadOnly ImageData.FileSubject = dso.SummaryProperties.Subject '* Requires dso.DLL' ImageData.FileTitle = dso.SummaryProperties.Title '* Requires dso.DLL' ImageData.FileType = FileType ImageData.FileWidth = SelectedImage.Width ImageData.FileWidthResolution = SelectedImage.HorizontalResolution Return True End Function 'ReadExistingData'
Just a couple of the "Top Box" search hits I've reviewed:
The dso.DLL: Very Helpful, but undesirable. Requires external DLL.
[http://]www.developerfusion.com/code/5093/retrieving-the-summary-properties-of-a-file/Incomplete Data ~ Does not answer my questions
[http://]msdn.microsoft.com/en-us/library/xddt0dz7.aspxRequires external DLL
[http://]www.codeproject.com/KB/GDI-plus/ImageInfo.aspxExternal Software required
[http://]stackoverflow.com/questions/3313474/write-metadata-to-png-image-in-netOld Data ~ Visual Studio 2005 and .NET 2.0
[http://]www.codeproject.com/KB/graphics/MetaDataAccess.aspxConvert to BMP: Looks useful
[http://]www.freevbcode.com/ShowCode.Asp?ID=5799