VBA Internet Explorer Automation 'Permission Denied'
Solution 1
After testing all above suggested fixes and even more, I am positive this is a weird bug with Eventhandling of child Processes invoked by IE whenever there are different security zones on one website.
Cut to the chase, here is the solution:
'Craziest workaround ever due to bugged IE Eventhandling
Do While IE.ReadyState = 4: DoEvents: Loop
Do Until IE.ReadyState = 4: DoEvents: Loop
Add this code piece every time after navigating to a new page or other events that might cause the website to reload, like clicking on a submit button or similar.
Dan above suggested to add DoEvents statements liberally in your code, which did not 100% do the trick for me. Instead, I suggest to add above code whereever applicable.
Kudos to Dan, without your comment I would've never figured it out!
Short summary of the BENEFITS this method has over other suggested fixes:
- You can stick to late binding if you wish (Dim IE as Object)
- You can stick creating a InternetExplorer object (as opposed to i.e. InternetExplorerMedium)
- You don't need to alter the security zone settings in IE
- No unnecessary wait times in your application
- You get rid of Error 70 - Permission denied
- You get rid of lost sessions i.e. "Run-time error '-2147417848 (80010108)': Automation error The object invoked has disconnected from its clients."
- You get rid of interface issues i.e. "Run-time error '-2147023179 (800706b5)': Automation error The interface is unknown"
Summary of all the things YOU DO NOT NEED with this approach:
- InternetExplorerMedium objects
- Early binding in general
- Reference to Microsoft Internet Controls
- Reference to Microsoft Shell Controls and Automation
- Weird error handling
- No need to ever check IE.Busy in your code
- Application.wait or sleep causing unnecessary guesstimated delays
I'm only including above summary because I've seen all those workarounds suggested and have personally tried them with no luck so far.
Example code based on OP's question:
Dim IE as Object
Set IE = CreateObject("InternetExplorer.Application")
IE.Visible = True
IE.Navigate("http://www.google.com")
'Craziest workaround ever due to bugged IE Eventhandling
Do While IE.ReadyState = 4: DoEvents: Loop
Do Until IE.ReadyState = 4: DoEvents: Loop
IE.document.getElementsByTagName("Input")(3).Value = "Search Term"
IE.document.Forms(0).Submit ' this previously crashed
I really hope this will help others in the future. Godspeed to everyone who helped with piecing the solution together.
Best Regards Patrick
Solution 2
I was having this exact same problem. Had a script for automating IE that had been working fine for a year. Then out of the blue I started receiving this "permission denied error". I did two things, one of which fixed the problem, but I don't know which one.
I removed the reference to "Microsoft Internet Controls", attempted to compile the project, added the reference to "Internet Controls" back in, and compiled the project.
Removed the 'WithEvents' keyword when declaring the InternetExplorer object since I did not need to capture events events from IE anyway.
I suspect it was the first and that somehow the reference became corrupted and removing at and re-adding it fixed the problem.
Solution 3
The solution of resetting the HTMLDocument Object works for me too i.e.
'After Page Loads, Reset HTMLDocument Object and Wait 1 Second Before recreating It
Set html = Nothing
Application.Wait Now + TimeValue("0:00:01") '<<< This seems to really help
Set html = IE.document
None of the other solutions have solved my problem.
Solution 4
I am receiving the same error when automating IE, but instead of fixing, I worked around.
Label1:
If myIe Is Nothing Then
Set myIe = CreateObject("InternetExplorer.Application")
End If
myIe.Navigate URL:=imdbLink
For Each link In myIe.document.Links
If Right(link.href, 9) = "/keywords" And Left(link.href, 26) = "http://www.imdb.com/title/" Then
mKPlot = link.href 'ERROR GENERATES FROM THIS, SOMETIMES...
End If
next link
errHandler:
If Err.Number = 70 Then
Debug.Print "permission denied for: |" & title & "|"
myIe.Quit
Set myIe = Nothing
Application.Wait Now + TimeValue("00:00:10")
Resume Label1:
End If
Not the sleek registry-fix you were imagining, but it works for me.
Solution 5
This is a common issue with Internet Explorer automation in VBA. It generally seems to happen after a new page has been loaded. I have had success using a combination of DoEvents, Application.Wait, and setting my HTMLDocument object to Nothing after a new page load.
I have posed sample code below to demonstrate:
Option Explicit
Sub IEAutomation()
Dim IE As InternetExplorer
Dim html As HTMLDocument
Dim i As HTMLHtmlElement
Dim search_bar_id As String
Dim submit_button_name As String
Dim search_term As String
'Define Variables
search_bar_id = "lst-ib"
submit_button_name = "btnK"
search_term = "Learning VBA"
'Create IE and Naviagate to Google
Set IE = CreateObject("InternetExplorer.Application") 'Late Binding
IE.Visible = True
IE.navigate "http://www.google.com"
'Wait for IE To Load
Do While IE.readyState <> READYSTATE_COMPLETE: DoEvents: Loop
'Create HTMLDocument Object
Set html = IE.document
'Enter Search Term into Search Bar and Click Submit Button
html.getElementById(search_bar_id).innerText = search_term
Application.Wait Now + TimeValue("0:00:01")
html.getElementsByName(submit_button_name)(, 1).Click
'Wait for New Page to Load (** DoEvents Helps with the Permission Issue **)
Do While IE.readyState <> READYSTATE_COMPLETE: DoEvents: Loop
'After Page Loads, Reset HTMLDocument Object and Wait 1 Second Before Recreating It
Set html = Nothing
Application.Wait Now + TimeValue("0:00:01") '<<< This seems to really help
Set html = IE.document
'Print All Elements In H3 Tags to Immediate Window in VBE
For Each i In html.getElementsByTagName("H3")
Debug.Print i.innerText
Next i
End Sub
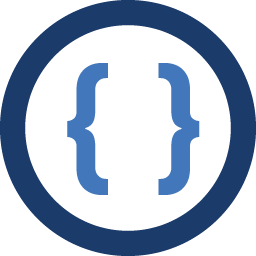
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Dim IE as New InternetExplorer IE.Visible = True IE.Navigate("http://www.google.com") Do Until IE.Busy = False Loop IE.document.getElementsByTagName("Input")(3).Value = "Search Term" IE.document.Forms(0).Submit <------ This line results in error.
The error states Run-time error 70: 'Permission Denied'
Please do not suggest code alterations. There is NOTHING wrong with the code. This macro works on 9 out of 10 computers. It is NOT a timing issue (I still get the error even if I step through manually). I know there are other ways to declare the internet explorer object. I have tried using CreateObject and all that stuff. None of it matters. Running as administrator does not help either.
This is just a simple example of the problem (we are actually automating much more complex tasks). So please do not ask "why do you want to do a goodle search?" and please do not ask "what are you trying to do". I need this problem solved. I don't need my code re written.
We use Windows XP, Internet Explorer 7, and Office 2003. Something is causing random people to not be able to automate internet explorer. It is not a user issue, but a computer issue. What I mean is on the culprit computers nobody can automate no matter which user logs in. But the same user can use a different computer and everything is fine. Therefore, it is likely a registry setting on the local machine or something like that. All computers are set up the same way here, same specs, same software.
I have googled and googled and googled and googled. Unfortunately run-time error 70 seems to be a catch all and a lot of users report the error for different symptoms. In my case I have not found a solution otherwise I would not be asking here.
The only way we can solve it is to have IT completely reload everything on the hard drive. A clean refresh including the operating system. That takes care of the problem but it also forces the user set their machine up again to the way they had it before and reinstall all of the software and everything. That is not a good solution. There is a setting somewhere on the machine causing this or the refresh would not have an effect. I want to know what that setting is (my feeling is it is a registry setting).
Any help is appreciated, thanks.