VBSCRIPT arrays keys
Solution 1
In addition to Andrew's solution on this page, I've been able to use the Dictionary object in VBScript to do what I was after using a syntax more like Associative Arrays normall use in other languages:
Dim result
Set result = CreateObject("scripting.dictionary")
result("age") = 60
result("firstname") = "Tony"
result("height") = 187
result("weight") = 76
msgbox("Age: " & result("age") & vbCr &_
"First Name: " & result("firstname") & vbCr &_
"Height: " & result("height") & vbCr &_
"Weight: " & result("weight") )
More information on the dictionary object can be found here: http://www.microsoft.com/technet/scriptcenter/guide/sas_scr_ildk.mspx
I hope that's helpful to others trying to do the same thing.
Thanks to Richard Mueller for providing this solution via the microsoft.public.scripting.vbscript Usenet group.
Cheers
Turgs
Solution 2
In order to acheive this, you need to use a Dictionary object from the Scripting library:-
Dim result : Set result = CreateObject("Scripting.Dictionary")
result.Add "Age", 60
result.Add "Name", "Tony"
and so on. You can retrieve items as:-
Dim age : age = result("Age")
However, if you have fixed set of identifiers you might consider defining a class:-
Class CResult
Public Age
Public Name
End Class
Dim result : Set result = new CResult
result.Age = 60
result.Name = "Tony"
MsgBox "Age: " & result.Age & vbCrLf & _
"Name: " & result.Nname) & vbCrLf
By the way, typically, we use CR LF for new lines not just CR. Also, if you are using a method or function as a statement (as in the MsgBox above) don't enclose parameters in ( ).
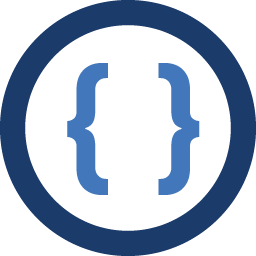
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I'm fairly new to vbscript and I'm struggling a little as I'm more familiar with Javascript. Can someone please give me a hand?
Does vbscript allow the use of named array keys like I have in my example below?
For example I have:
Dim result(3) result(age) = 60 result(firstname) = "Tony" result(height) = 187 result(weight) = 76 msgbox("Age: " & result(age) & vbCr &_ "Name: " & result(firstname) & vbCr &_ "Height: " & result(height) & vbCr &_ "Weight: " & result(weight) )
The resulting msgbox shows:
Age: 76 Name: 76 Height: 76 Weight: 76
This seems to assign every element in the result array to 76, which should only be assigned to the "weight" element.
Is this happening because vbscript only accepts integers as the key/ index for arrays?
Any help is greatly appreciated.
Thanks Turgs
-
We Are All Monica over 14 yearsIncidentally, the reason you were getting 76 is because all of your "keys" are being interpreted as undefined variables, which are evaluated as zero with no warning.
-