Vertical menu bootstrap and angular
Solution 1
Just use CSS, apply different styles to your submenu when "noneStyle" is toggled, under normal style, your submenu should use position: absolute; left: --px;
for positioning, and under "noneStyle", it should be position: static
to force it display with initial style.
and BTW, please do not place <div>
tag under <ul>
, it's not a good way to write HTML, I've changed it for you.
var app = angular.module('myApp', []);
app.controller('mainCtrl', function ($scope) {
$scope.noneStyle = false;
$scope.bodyCon = false;
$scope.sasd = "asd";
//Toggle the styles
$scope.toggleStyle = function () {
//If they are true, they will become false
//and false will become true
$scope.bodyCon = !$scope.bodyCon;
$scope.noneStyle = !$scope.noneStyle;
}
//add class to search box
$scope.openSearch = false;
$scope.searchToggle = function () {
$scope.openSearch = !$scope.openSearch;
}
});
body{
background:#300F18 !important;
}
li{
list-style:none;
}
.rightbar {
background: none repeat scroll 0 0 #fff;
border-left: 5px solid #ccc;
height: 100%;
padding-top: 50px;
position: fixed;
right: 0;
width: 60px;
z-index: 200;
}
.rightbar ul li {
color: #333;
font-weight: 100;
padding: 16px 0 21px;
/*width: 0;*/
transition:all 0.3s ease;
-webkit-transition:all 0.3s ease;
-moz-transition:all 0.3s ease;
-o-transition:all 0.3s ease;
transition:all 0.3s ease;
}
.rightbar ul li:hover {
background:inherit;
width:100%;
}
.rightbar ul li a {
color: #8b8b8b;
float: right;
font-size: 12px;
line-height: 23px;
padding-right: 54px !important;
font-size:0;
transition: all 0.2s ease 0s;
-webkit-transition:all 0.1s ease;
-moz-transition:all 0.1s ease;
-o-transition:all 0.1s ease;
transition:all 0.1s ease;
}
.accordion-heading .accordion-toggle{
padding:0;
}
.rightbar ul li a i{
color: #8b8b8b;
float: right;
font-size: 22px;
position: absolute;
right: 15px;
transition:all 0.3s ease;
-webkit-transition:all 0.3s ease;
-moz-transition:all 0.3s ease;
-o-transition:all 0.3s ease;
transition:all 0.3s ease;
}
.accordion-group{
border:medium none;
}
.tools-menu {
position: relative;
}
.tools-menu .submenu {
position: absolute;
width: 100px;
left: -140px;
background-color: #fff;
display: none;
}
.tools-menu:hover .submenu {
display: block;
}
.noneStyle .tools-menu .submenu {
display: block;
position: static;
}
.accordion-inner ul li{
padding:10px 0;
list-style:none;
}
.rightbar ul li:hover a,
.rightbar ul li:hover a i {
color: #23b7e5;
text-decoration: none;
}
.noneStyle a{
font-size:13px !important;
}
.noneStyle {
width: 144px;
z-index: 1000;
transition:all 0.3s ease;
-webkit-transition:all 0.3s ease;
-moz-transition:all 0.3s ease;
-o-transition:all 0.3s ease;
transition:all 0.3s ease;
}
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" type="text/css" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap.min.css">
<div ng-app="myApp" ng-controller="mainCtrl">
<div class="navbar navbar-default topnavbar">
<ul class="nav navbar-nav">
<li>
<a href="#" ng-click="toggleStyle()">
<em class=" fa fa-navicon">
</em>
</a>
</li>
</ul>
</div>
<aside class="rightbar" id="rightMenu" ng-class="{'noneStyle' : noneStyle}">
<ul>
<li><a href="#/456">Dashboard<i class="fa fa-tachometer"></i></a></li>
<li><a href="#/123">write<i class="fa fa-pencil-square-o"></i></a></li>
<li><a href="#/963">list<i class="fa fa-list"></i></a></li>
<li class="tools-menu">
<a class="accordion-toggle" data-toggle="collapse" data-parent="#rightMenu" href="#collapseFour">
Tools<i class="fa fa-file-text"></i>
</a>
<ul class="submenu">
<li> 1</li>
<li> 2</li>
<li> 3</li>
</ul>
</li>
</ul>
</aside>
{{sasd}}
</div>
Solution 2
Try this. http://jsfiddle.net/zqdmny41/4/
Remove the collapse class from your sub menu list and use ng-mouseenter on the menu and ng-mouseleave on the aside tag.
<aside class="rightbar" id="rightMenu" ng-class="{'noneStyle' : noneStyle}" ng-mouseleave="subMenu = false">
<a class="accordion-toggle" data-toggle="collapse" data-parent="#rightMenu" href="#" ng-mouseover="subMenu = true">Tools<i class="fa fa-file-text"></i></a>
Also your div and li tags are all over the place, please structure them correctly. For example, div cannot come in between two li tags.
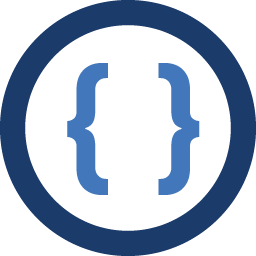
Admin
Updated on April 18, 2020Comments
-
Admin about 4 years
I'm trying to create vertical menu like this site . the thing i'm looking for is : when menu is collapse sub menus open with hover and when menu is open , sub menus open below the links .
Here is my JSFiddle , what i've done is : i can open the menu after click the button .
sub menu is working (last one) but i don't want to open submenu when menu is collapsed .Angular
var app = angular.module('myApp', []); app.controller('mainCtrl', function ($scope) { $scope.noneStyle = false; $scope.bodyCon = false; $scope.sasd = "asd"; //Toggle the styles $scope.toggleStyle = function () { //If they are true, they will become false //and false will become true $scope.bodyCon = !$scope.bodyCon; $scope.noneStyle = !$scope.noneStyle; } });
UPDATE
menu with submenu :
submenu open with hover
that's what i want !! thx in advance
-
Admin over 9 yearsthx @Aakash , but this is not what i want , i've udpdate my question, please check it out
-
Admin over 9 yearssure, i'll do it, but i dont want to submenu open with hover when menu is open, see my question and links
-
Admin over 9 yearsman you're the best and creative, but it's not the best and clean way. thx a lot
-
Aakash over 9 yearsYeah you'll have to still do a lil bit of fine tuning. Your welcome.