@Viewchild can not see matSort
Solution 1
It will be initialized and available in the AfterViewInit
lifecycle hook:
export class MyClassComponent implements AfterViewInit {
@ViewChild(MatSort) sort: MatSort;
ngAfterViewInit() {
console.log(this.sort); // MatSort{}
}
}
More info on lifecycle hooks here: https://angular.io/guide/lifecycle-hooks.
Solution 2
You have probably already imported at the class level with:
import { MatSort, MatTableDataSource } from '@angular/material';
This makes the types MatSort and MatTableDataSource available within your .ts class. However, you are also trying to use the related modules as directives in your component's .html file, to do this your app.module.ts needs to be importing them, I do this with a separate NgModule that imports all of the material components I use i.e.
material\material.module.ts
import { NgModule } from '@angular/core';
import {
MatTableModule,
MatSortModule,
} from '@angular/material';
@NgModule({
imports: [
MatTableModule,
MatSortModule,
],
exports: [
MatTableModule,
MatSortModule,
]
})
export class MaterialModule { }
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { HttpClientModule } from '@angular/common/http';
import { MaterialModule } from './material/material.module';
import { AppComponent } from './app.component';
import { myComponent } from './my/my.component';
@NgModule({
declarations: [
AppComponent,
MyComponent
],
imports: [
BrowserModule,
MaterialModule,
BrowserAnimationsModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Solution 3
I'm working with angular 7 what solved the issue for me was adding MatSortModule in the app.modules.ts. The error I got is the one mentioned here. No indication appeared about the MatSortModule being missing.
Original answer here: Angular 5 - Missing MatSortModule
Solution 4
In Angular 8 I had to use a setter and optional parameter static=false:
@ViewChild(MatSort, {static: false})
set sort(sort: MatSort) {
this.sortiments.sort = sort;
this.selectedSortiments.sort = sort;
}
I found the answer from here: https://stackoverflow.com/a/47519683/3437868 https://github.com/angular/components/issues/15008#issuecomment-516386055
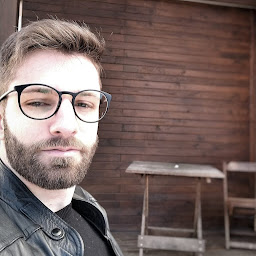
Luiz Ricardo Cardoso
Updated on June 09, 2022Comments
-
Luiz Ricardo Cardoso almost 2 years
In my Angular application, my @ViewChild instance is failing to fill HTL matSort.
mycomponent.ts:
import { MatSort } from '@angular/material'; export class MyClassComponent { @ViewChild(MatSort) sort: MatSort; } ngOnInit() { console.log(this.sort); // undefined }
mycomponent.html:
<mat-table #table [dataSource]="dataSource" matSort> ....................................................... ....................................................... </mat-table>
I need to use sortChange from matSort but it is always returned undefined.
-
Luiz Ricardo Cardoso about 6 yearsIt is not entering the ngAfterViewInit () method, I implemented AfterViewInit and created the method as it is in the documentation, but it does not enter the method when the page loads.
-
vince about 6 yearsIt was running
ngOnInit
but now it's not runningngAfterViewInit
? Check for typos and such...that behavior seems odd. -
Luiz Ricardo Cardoso about 6 years
ngOnInit
With this method this.sort comes undefined, but withngAfterViewInit
method... I can not know since neither is done the method call in execution... there are no typos -
Luiz Ricardo Cardoso about 6 yearsAfter several attempts, this is calling the
ngAfterViewInit ()
method, but within thisthis.sort
method remainsundefined
. -
Luiz Ricardo Cardoso about 6 yearsAnother problem is also that
mat-sort-header
has no effect on the table, only@ViewChild matPaginator
is returned. -
mminnie almost 6 yearsI have the same issue. :-/
-
mminnie almost 6 yearsI had the same issue.....but mine was related to a *ngIf. See this answer https://stackoverflow.com/a/48322035/2022096
-
mminnie almost 6 yearsI thought it was fixed. Ultimately it ended up being I needed to import the MatSortModule into the @NgModule. I was importing MatSortModule in my component but not into the NgModule.
-
NJS over 2 yearsIn my case, setting the ViewChild parameter
{static: true}
ensures the property has a value when I access it inngOnChanges()
. Your answer pointed me in the right direction -
Dai over 2 years