VM1374:32 Uncaught TypeError: Converting circular structure to JSON(…)
This is a pretty common problem. Converting circular structure to JSON(...)
is thrown because you're trying to print out an object that eventually references itself through one of its properties.
Here's a JSFiddle of one of the simplest ways you can get this issue:
var a = {
b: null
};
// Prints fine
console.log(JSON.stringify(a, null, 3));
a.b = a;
// Throws an error, as a.b *is* a
console.log(JSON.stringify(a, null, 3));
When you call JSON.stringify
, the browser will traverse your object like a big tree, going down each property like a branch and converting what it can into a string.
In the example above, the property b
at first is null, which results in a successful "stringification". When we set a.b
to a
itself, we now get this sort of tree:
a
|-b: a
|-b: a
|-b: a
...
The structure is circular in this way because the object references itself. There's no way to write that as JSON because it would go on forever, so an error is thrown.
For your specific problem, this is happening in React because the React objects reference their parents, which reference their children, which reference their parents, which reference their children... it goes on forever.
So you can't use JSON.stringify
on this
or this.props
in your example for this reason (this.props
has the property children
that is partly responsible for the issue).
You will notice that you can stringify this.state
, as that's a normal object that doesn't reference any other React objects:
> JSON.stringify(this.state);
"{"selected":0}"
Edit: What may be best for you is to just console.log
without JSON.stringify
:
console.log("this.props.children[this.state.selected]---->", this.props.children[this.state.selected]);
You can add multiple arguments to console.log
and separate them with commas, then the browser console itself should print it in a viewable format.
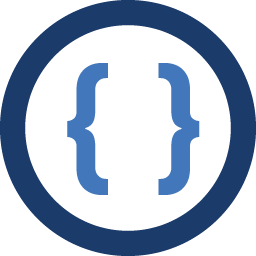
Admin
Updated on June 17, 2022Comments
-
Admin almost 2 years
I am new to JS. I am trying to print the values in my console.log. I am getting the below error:
VM1374:32 Uncaught TypeError: Converting circular structure to JSON(…)
My code is below:
console.log("this.props.children[this.state.selected]---->" + JSON.stringify(this.props.children[this.state.selected]));