Vue.js - Add or set new data to object in store
13,215
Solution 1
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
// userData: {}
userData: []
},
mutations: {
ADD_USER_DATA: (state, data) => {
state.userData.push(data)
}
}
})
You are trying to use a push
method of Object. Object does not have a push method you should initiate userData
value with Array []
or assign that data to the object
Solution 2
if you need reactivity:
mutations: {
ADD_USER_DATA: (state, data) => {
Object.keys(data).forEach( key => {
Vue.set(state.userData, key, data[key])
})
}
Solution 3
I've ended up using Object.assign
method.
mutations: {
ADD_USER_DATA: (state, data) => {
// state.userData.assign(data)
state.userData = Object.assign({}, data)
}
}
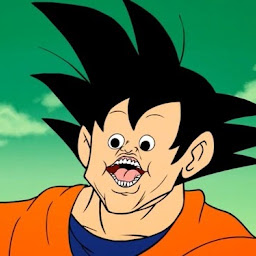
Author by
Vincent Von
Updated on June 17, 2022Comments
-
Vincent Von almost 2 years
I'm trying to add or update an object to store with Vuex.
import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state: { userData: {} }, mutations: { ADD_USER_DATA: (state, data) => { state.userData.push(data) } } })
This returns
state.userData.push
is not a function.And in the components:
<template> <div> <input type="date" v-model="inputData.date1"> <input type="date" v-model="inputData.date2"> <input type="number" v-model="inputData.date3"> <button @click="submitForm">Submit</button> </div> </template> <script> import { mapState, mapMutations } from 'vuex' export default { data () { return { inputData: {} } }, computed: { ...mapState([ 'userData' ]) }, methods: { ...mapMutations([ 'ADD_USER_DATA' ]), submitForm () { this.ADD_USER_DATA(this.inputData) } } } </script>
Later on I want to update
userData
with a value from other component, so that it impacts both components. I would like to have a nice way of adding, replacing, concating the old array with a new array. I followed the example in this video.(FYI: I'm currently learning Vue.js from scratch and couldn't figure out this Vuex's mutations, actions...)
-
Vincent Von over 5 yearsBut with Array I can push multiple arrays to it. I'm simply trying to store an Object in state.
-
Peyman Majidi almost 3 yearsthanks for answering, please add some comments and note to make it more clear
-
jasie almost 3 yearsThank you for this code snippet, which might provide some limited, immediate help. A proper explanation would greatly improve its long-term value by showing why this is a good solution to the problem and would make it more useful to future readers with other, similar questions. Please edit your answer to add some explanation, including the assumptions you’ve made.