Vue.js webpack : how to get the list of files in a directory?
Solution 1
fs
is server side module. You cannot use fs
in the browser, there is no solution to this, it's technically impossible.
https://github.com/vuejs-templates/webpack/issues/262
Solution 2
You can utilize webpack's require.context() to list files in a given directory at compile time.
https://webpack.js.org/guides/dependency-management/
const illustrations = require.context(
'@/assets/illustrations',
true,
/^.*\.jpg$/
)
console.log(illustrations.keys())
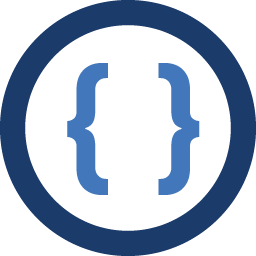
Admin
Updated on June 09, 2022Comments
-
Admin about 2 years
I am trying to get a list of all files in the 'assets/illustrations' directory of a SPA static app as a JSON object to be re-used in my Vuex store
the assets/illustrations dir structure is :
assets illustrations ill-1 prop-1-1.jpg prop-1_2.jpg ill-2 prop-2-1.jpg prop-2_2.jpg ill-3 prop-3-1.jpg prop-3_2.jpg
my target is to have such object as a result :
{ illustrations: [ill-1: [prop-1-1.jpg, prop-1_2.jpg], ill-2: [prop-2-1.jpg, prop-2_2.jpg], ill-3: [prop-3-1.jpg, prop-3_2.jpg]] }
In my App.vue , I fire a method getIllustrations() when the app component is mounted
<script> export default { name: 'app', data () { return {} }, methods: { getIllustrations () { const path = require('path') const fs = require('fs') fs.readdir(path.join(__dirname, 'assets/illustrations'), (err, items) => { if (err) { console.log(err) } console.log(items) }) } }, mounted () { this.getIllustrations() } } </script>
but I get an error :
Error in mounted hook: "TypeError: fs.readdir is not a function"
I also tried to run this function in the main.js file ( which is running on the server ..) same error..
WHere am I wrong ? thanks for feedback
-
HJo almost 5 yearsNot true, you can use require.context()
-
giuliano-oliveira over 3 yearsBe aware that for custom file formats (like yaml) you need a custom loader for this to work without warnings, and i think that with some loaders + regex it will increase your bundle size / memory consumption as stated in the docs.