Vue - Using component inside other component
Solution 1
By default you have to use it with kebab-case in template
tag.
Register component as of PascalCase notation. Use it in component with kebab-case notation. Simple as that!
Case 1 : Default
<template>
<section class="home">
<div class="hero-content">
<h1>Hello World</h1>
<search-bar></search-bar>
</div>
</section>
</template>
<script>
import SearchBar from './common/SearchBar'
export default{
components: SearchBar
}
</script>
Case 2 : Customized
Or you want to register it with your way(by applying custom name) just change this code :
export default{
components: {
'search-bar' : SearchBar
}
}
UPDATE: This answer was provided in reference to vue version 2.x. I am unaware of vue 3.x and haven't read 3.x docs. You can always submit an edit draft for vue 3.x compatible solution. Thanks!
Solution 2
Script of Home.vue should be like following
import SearchBar from './common/SearchBar'
export default {
name: 'Home',
components: {
'SearchBar': SearchBar
}
}
If you SearchBar component already have a name property values SearchBar, the 'SearchBar' filed name is not required.
Solution 3
May or May not help you, but I did a silly mistake: as
import ServiceLineItem from "@/Pages/Booking/ServiceLineItem";
export default {
components: ServiceLineItem,
};
As it should be like :
import ServiceLineItem from "@/Pages/Booking/ServiceLineItem";
export default {
components: {
ServiceLineItem,
},
};
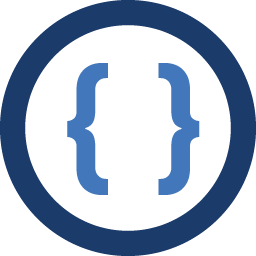
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
I am trying to use a
search-bar component
inside myhome.vue
component but I can't figure out why its not working.Folder structure
App.vue components home.vue query.vue common HeaderBar.vue SideBar.vue SearchBar.vue
App.vue
script
import HeaderBar from './components/common/HeaderBar' import SideBar from './components/common/SideBar' export default { name: 'App', components: { HeaderBar, SideBar },
html
<template> <div id="app"> <HeaderBar></HeaderBar> <SideBar></SideBar> <main class="view"> <router-view></router-view> </main> </div> </template>
This above works just fine.
Home.vue
script
import SearchBar from './common/SearchBar' export default { name: 'Home', components: SearchBar }
html
<template> <section class="home"> <div class="hero-content"> <h1>Hello World</h1> <SearchBar></SearchBar> </div> </section> </template>
I get those errors:
[Vue warn]: Invalid component name: "_compiled". Component names can only contain alphanumeric characters and the hyphen, and must start with a letter.
[Vue warn]: Invalid component name: "__file". Component names can only contain alphanumeric characters and the hyphen, and must start with a letter.
[Vue warn]: Unknown custom element: - did you register the component correctly? For recursive components, make sure to provide the "name" option.
found in
---> <Home> at src\components\Home.vue <App> at src\App.vue <Root>
-
timbrown over 4 yearsEdited answer - This is camelCase. This is PascalCase.