waiting thread until a condition has been occurred
Solution 1
You need conditional variables.
If your compiler supports std::conditional
introduced by C++11, then you can see this for detail:
If your compiler doesn't support it, and you work with win32 threads, then see this:
And here is a complete example.
And if you work with POSIX threads, then see this:
You can see my implementation of conditional_variable
using win32 primitives here:
Scroll down and see it's implementation first, then see the usage in the concurrent queue implementation.
A typical usage of conditional variable is this:
//lock the mutex first!
scoped_lock myLock(myMutex);
//wait till a condition is met
myConditionalVariable.wait(myLock, CheckCondition);
//Execute this code only if the condition is met
whereCheckCondition
is a function (or functor) which checks the condition. It is called by wait()
function internally when it spuriously wakes up and if the condition has not met yet, the wait()
function sleeps again. Before going to sleep, wait()
releases the mutex, atomically.
Solution 2
If you don't have C++11, but you do have a system that supports POSIX threads, then you can use a condition variable. There are other choices, but a condition variable may be the most straight forward given the way you have described your problem.
A pthread condition variable is used in conjunction with a mutex. The trick with the condition variable is that waiting on it causes the acquired mutex to be released, until the wait call returns, at which point the mutex has been acquired again. The sequence is:
- acquire mutex
- while PREDICATE is not true
- wait on condition variable
- do work on critical section
- if PREDICATE is true
- signal condition variable
- release mutex
The signal step is used in case multiple threads are entering the same critical section above.
If a different thread may access the same mutex to modify state that affects the PREDICATE, that thread should check to see if anyone needs to be signaled.
- acquire mutex
- do work on critical section
- if PREDICATE is true
- signal condition variable
- release mutex
The POSIX commands of interest are:
pthread_mutex_init()
pthread_mutex_destroy()
pthread_mutex_lock()
pthread_mutex_unlock()
pthread_cond_init()
pthread_cond_destroy()
pthread_cond_wait()
pthread_cond_signal()
Solution 3
Using Semaphore for signalling. Example (application clean exit) as below:
Declare in header
static sem_t semPrepareExit; //declaration
In source (main thread);
sem_init(&semPrepareExit, 0, 0); ///semaphore initialized
...
///now wait for the signal on the semaphore, to proceed hereforth
sem_post(&semPrepareExit);
/// cleanup ahead
...
In source, (spawned-thread);
...
sem_post(&semPrepareExit);
Now, as soon as you signal on the semaphore using "sem_post". The main-thread will receive the signal at the wait-node/point, and will proceed, there-forth.
Solution 4
try something like this :
class CmyClass
{
boost::mutex mtxEventWait;
bool WaitForEvent(long milliseconds);
boost::condition cndSignalEvent;
};
bool CmyClass::WaitForEvent(long milliseconds)
{
boost::mutex::scoped_lock mtxWaitLock(mtxEventWait);
boost::posix_time::time_duration wait_duration = boost::posix_time::milliseconds(milliseconds);
boost::system_time const timeout=boost::get_system_time()+wait_duration;
return cndSignalEvent.timed_wait(mtxEventWait,timeout); // wait until signal Event
}
// so inorder to wait then call the WaitForEvent method
WaitForEvent(1000); // it will timeout after 1 second
// this is how an event could be signaled:
cndSignalEvent.notify_one();
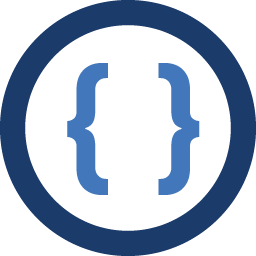
Admin
Updated on December 01, 2020Comments
-
Admin over 3 years
I want to wait one thread of 2 thread that executed in a Simultaneous simulator until a condition has been occurred, may be the condition occurred after 1000 or more cycles of running a program in the simulator, after the condition occurred the waited thread executed again, how can I do it?