Want to remove the time from the DataTable Date column
11,932
Solution 1
rowString += "\"" + DateTime.Parse(dt.Rows[x][y]).ToString("dd/MM/yyyy") + "\"";
or fully implemented like:
DataTable dt = TypeConveriontHelper.ConvretExcelToDataTable(SourceAppFilePath);
string targetPath = BatchFolderPath + @"\" + "TRANSACT.INX";
StreamWriter wrtr = null;
wrtr = new StreamWriter(targetPath);
for (int x = 0; x < dt.Rows.Count; x++)
{
string rowString = "";
for (int y = 0; y < dt.Columns.Count; y++)
{
string dateTimeString = dt.Rows[x][y].ToString();
DateTime dateTime;
if (DateTime.TryParse(dateTimeString, out dateTime))
{
string formattedDate = dateTime.ToString("dd/MM/yyyy");
if (y == dt.Columns.Count - 1)
{
rowString += "\"" + formattedDate + "\"";
}
else
{
rowString += "\"" + formattedDate + "\"~";
}
}
}
rowString = rowString.Replace("\"", String.Empty).Trim();
wrtr.WriteLine(rowString);
}
wrtr.Close();
wrtr.Dispose();
like michele said see http://msdn.microsoft.com/en-us/library/az4se3k1.aspx for full reference
Solution 2
DateTime dateAndTime = DateTime.Parse(string);
DateTime dateOnly = dateAndTime.Date;
String dateString = dateAndTime.ToShortDateString();
or Custom Date and Time Format
dateAndTime.ToString("MMMM dd, yyyy");
For your code:
StringBuilder rowString = new StringBuilder();
int dateTimeColumn = 3;
for (int y = 0; y < dt.Columns.Count; y++)
{
string cellValue = dt.Rows[x][y].ToString();
if(y == dateTimeColumn)
{
DateTime dateAndTime = DateTime.Parse(cellValue);
cellValue = dateAndTime.ToShortDateString();
}
rowString.Append(cellValue);
if (y != dt.Columns.Count - 1)
{
rowString.Append("~");
}
}
wrtr.WriteLine(rowString.ToString());
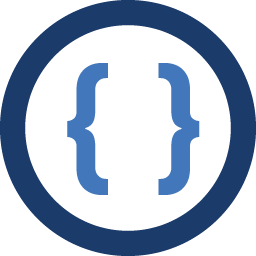
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
DataTable dt = TypeConveriontHelper.ConvretExcelToDataTable(SourceAppFilePath); string targetPath = BatchFolderPath + @"\" + "TRANSACT.INX"; StreamWriter wrtr = null; wrtr = new StreamWriter(targetPath); for (int x = 0; x < dt.Rows.Count; x++) { string rowString = ""; for (int y = 0; y < dt.Columns.Count; y++) { if (y == dt.Columns.Count - 1) { rowString += "\"" + dt.Rows[x][y].ToString() + "\""; } else { rowString += "\"" + dt.Rows[x][y].ToString() + "\"~"; } } rowString = rowString.Replace("\"", String.Empty).Trim(); wrtr.WriteLine(rowString); } wrtr.Close(); wrtr.Dispose();
Datatable returns "Date" column which includes Time along with Date. But I need to display only the date. Provide me a solution. Thanks.
-
Admin over 11 yearsHow I have to use the datetime? Whether I need to put inside the for loop. Please help. Thanks.