Warning C4800: 'int' : forcing value to bool 'true' or 'false' (performance warning)
30,991
Solution 1
FYI: Casts won't hide the warning by design.
Something like
return (id & MID_NUMBER) != 0;
should clearly state "I want to check whether this value is zero or not" and let the compiler be happy
Solution 2
Where's the declaration of id and MID_NUMBER? Are you sure they are not windef-style BOOLs rather than (lowercase) bool's? BOOL's have been in windef for decades typedef'd as an int; they pre-date proper C++ bool's and a lot of developers still use them.
Solution 3
Use the !! idiom eg
bool CBase::isNumber()
{
return !!(id & MID_NUMBER);
}
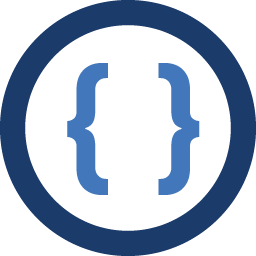
Author by
Admin
Updated on September 22, 2020Comments
-
Admin almost 4 years
I have this problem in my code:
bool CBase::isNumber() { return (id & MID_NUMBER); } bool CBase::isVar() { return (id & MID_VARIABLE); } bool CBase::isSymbol() { return (id & MID_SYMBOL); }
-
Admin over 10 yearsJust to please the compiler !??
-
deviantfan over 10 yearsAnd why 2 ops instead of 1 (even if it is optimized)?
-
deviantfan over 10 years@Dieter Lücking: Non-standard workarounds are probably worse.
-
Admin over 10 years@deviantfan The warning itself is useless
-
CreativeMind over 10 years@DieterLucking: It can not be useless as it is warning.
-
deviantfan over 10 years@Lücking: Why? Of course the compiler can generate the correct stuff without changed code, but maybe the coder has not intended a implicit cast and finds a semantic error because of the warning?
-
cup over 10 yearsFirst inverts it so you get the inverse of what you actually wanted, second inverts that so you get what you actually wanted. Useful when you have a mix of BOOL (windows), Bool (xwindows) and bool (C++)
-
ildjarn over 10 years@deviantfan : The warning is not about semantics, it is about performance, which is specious.
-
André Bergner about 7 yearsI give you a +1. This was useful for me, since I have this warning in templated code where I cannot compare against 0 but !! works.